405 method not allowed using Tomcat
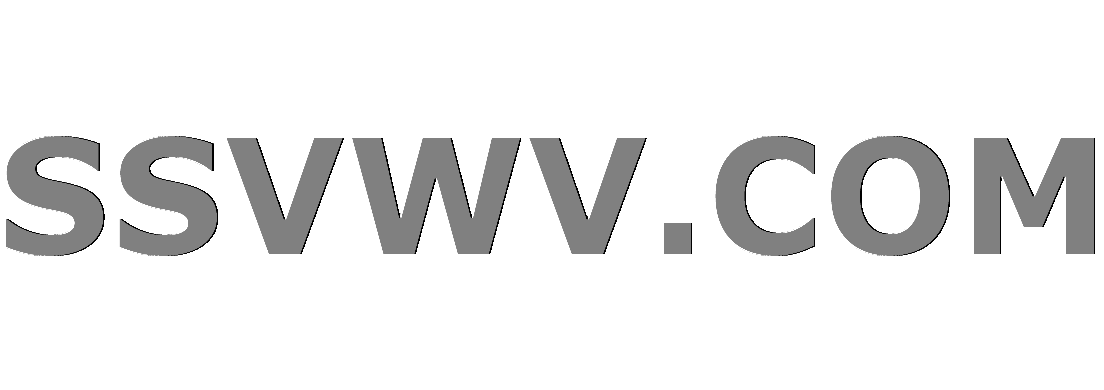
Multi tool use
405 method not allowed using Tomcat
I am new to web development and having this issue for some time. I tried a lot of things that were suggested on SO and elsewhere, but no luck. Anyways, i'll explain what I am doing.
I am trying a simple exercise where I have a simple HTML page that accepts a city name and on clicking the submit button, will show me the weather of that city. The HTML code is as below:
<body>
<form>
<div>
<label for="city">City</label>
<input id="city" type="text" name="city">
</div>
<div>
<input id="submitButton" type="button" name="submitButton" value="Get weather!" onclick="send()">
</div>
</form>
<script type="text/javascript" src="CityWeather.js"></script>
</body>
This HTML file makes a call to my JS script which will handle the call to the API of OpenWeatherMap and display the results. Code for the JS is as below(please note I have purposely omitted the api_key but the actual URL does have it):
function send() {
var xhttp = new XMLHttpRequest();
xhttp.open("GET", "http://api.openweathermap.org/data/2.5/weather?q=london&APPID=<api_key>", true);
xhttp.setRequestHeader("Content-type", "application/json");
xhttp.send();
var jsonResponseText = xhttp.responseText;
//var response = JSON.parse(jsonResponseText);
console.log(xhttp.readyState);
console.log(xhttp.status);
console.log(jsonResponseText);
}
Now, when I first tried obtaining the results by just launching the HTML file, I got CORS issue and I realized that I should not be making the call to the API via the file URL(file://). So I downloaded Tomcat 9.0 and placed both, the HTML and JS files, inside the webapps folder of Tomcat. I then started Tomcat and launched the page. However, I get the exceptions mentioned below:
OPTIONS http://api.openweathermap.org/data/2.5/weather?q=london&APPID=<api_key> 405 (Method Not Allowed)
Failed to load http://api.openweathermap.org/data/2.5/weather?q=london&APPID=<api_key>: Response for preflight has invalid HTTP status code 405.
I tried updating the CATALINA_BASE/conf/web.xml file with the entries below,
<filter>
<filter-name>CorsFilter</filter-name>
<filter-class>org.apache.catalina.filters.CorsFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>CorsFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
restarted the server and tried it again but still no luck. Can someone help me by pointing what needs to be done? I am using Chrome for this exercise. I tried using IE as well but no luck.
UPDATE 1:
I tried changing the api call. Instead of using the openweathermap api, I tried using the GIT API to get my repos.
function send() {
var xhttp = new XMLHttpRequest();
xhttp.open("GET", "https://api.github.com/users/bhavyas66", true);
xhttp.setRequestHeader("Content-type", "application/json");
xhttp.send();
var jsonResponseText = xhttp.responseText;
//var response = JSON.parse(jsonResponseText);
console.log(xhttp.readyState);
console.log(xhttp.status);
console.log(jsonResponseText);
}
In this case, I didnt get the exceptions above, but the state is stuck at 1 and status printed is 0. The response is also empty. However, when I open the Chrome Network window, I can see that there is response under the Preview tab
It doesn’t matter what CORS config you do on your own server. See the comment at stackoverflow.com/questions/50518345/…. You must do the request from your backend instead. You’re making a request to the openweathermap.org API, which doesn’t handle the CORS preflight OPTIONS request right. And if when make requests to that API, you add a 'Content-Type: application/json' header to the request, that triggers browsers to send a CORS preflight OPTIONS request, which that API doesn’t handle
– sideshowbarker
Jul 2 at 13:34
1 Answer
1
try "POST" method,405 errors often appear at the same time as the POST method
I tried that as well but no luck.
– BoJack Horseman
Jul 2 at 4:38
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Why are you adding a 'Content-type: application/json' header to a GET request? Adding that header to the request has no effect other than to trigger browsers to make a CORS preflight OPTIONS request. Remove that header from your request.
– sideshowbarker
Jul 2 at 13:28