Correct way to use AutoMapper in ASP.Net MVC
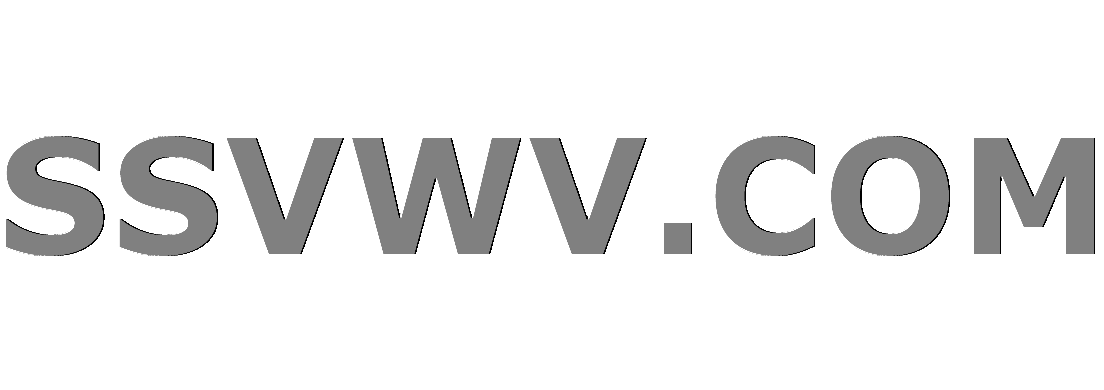
Multi tool use
Correct way to use AutoMapper in ASP.Net MVC
I'm trying to start using ViewModels - but I'm having trouble with this POST not validating - the values in the model are shown in the Watch part below the code:
ModelStats.IsValid = false
My ItemViewModel is:
public class ItemViewModel
{
public int ItemId { get; set; }
[Display(Name = "Item")]
public string ItemName { get; set; }
[Display(Name = "Description")]
public string Description { get; set; }
[Display(Name = "Price")]
public double UnitPrice { get; set; }
[Range(0.00, 100, ErrorMessage = "VAT must be a % between 0 and 100")]
public decimal VAT { get; set; }
[Required]
public string UserName { get; set; }
}
I'm sure it will be something simple - but I've just been looking at it so long, I can't figure out what I'm doing wrong. Can anyone please advise?
Thanks, Mark
UserName
2 Answers
2
As far as Validation failure is concerned.
If you are not supplying UserName
in the form, then remove [Required] from ItemViewModel
UserName
ItemViewModel
In order to Use AutoMapper
First, Create a map, such as
Mapper.CreateMap<Item, ItemViewModel>();
And then map
var itemModel = Mapper.Map<Item, ItemViewModel>(model);
Refer: How do I use AutoMapper? section in https://github.com/AutoMapper/AutoMapper/wiki/Getting-started
Hi - thanks - it's the ModelState which is not validating - so not getting as far as the mapper. Can you see anything wrong with that? Thanks, Mark
– Mark
May 10 '13 at 8:00
@fixit, If
ModelState
is not valid then you have put some validation which are failing, ModelState
has nothing to do with AutoMapper– Satpal
May 10 '13 at 8:02
ModelState
ModelState
You have to notice that, CreateMap has to be created once. You should not create it every request.
– Mariusz
May 10 '13 at 9:18
@stom, Yes that's correct
– Satpal
Jan 14 '16 at 13:12
Yes you should register it @ start up only
– Mariusz
Jan 14 '16 at 13:13
Make sure your ItemViewModel
, Item
classes have same fields or not. If same fields with same Datatypes AutoMapper works fine.
ItemViewModel
Item
Mapper.CreateMap< Item, ItemViewModel>();
Mapper.Map< Item, ItemViewModel>(ItemVM);
If Fields are not same in both the classes make sure that same with Custom Mapping.
Mapper.CreateMap<UserDM, UserVM>().ForMember(emp => emp.Fullname,
map => map.MapFrom(p => p.FirstName + " " + p.LastName));
In the above Custom Mapping Fullname
is UserVM
field that maps with FirstName
, LastName
fields from UserDM
(here UserDM
is Domain Model, UserVM
is View Model).
Fullname
UserVM
FirstName
LastName
UserDM
UserDM
UserVM
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
The validation of the ViewModel is before you set the
UserName
property, so, I guess, it's null and the validation fails. Why do you need a required user name in your ViewModel anyway?– Zabavsky
May 10 '13 at 8:00