Javascript new array prototype in new object constructor
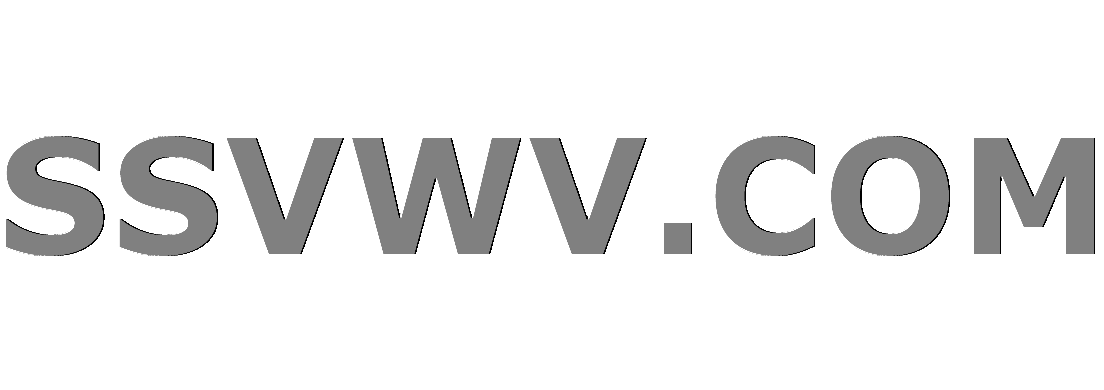
Multi tool use
Javascript new array prototype in new object constructor
If I do:
Array.prototype.test = "test"
Array.prototype.t = function() {return "hello"}
Every new Array
will have the property test
and the method t
.
Array
test
t
How can I do the same without affecting all Arrays?
Like:
Names = function(arr){
// Contacts constructor must be the same of Array
// but add the property test and the function t
}
z=new Names(["john","andrew"])
So that z.test
will return "test"
and z.t()
will return "hello"
?
(but Array.test
and Array.t
would stay undefined
)
z.test
"test"
z.t()
"hello"
Array.test
Array.t
undefined
I explain better:
Array.prototype.t="test";
Array.prototype.test = function(){ return "hello";}
z=new Array("john", "andrew")
console.log(z);
But this affects ALL arrays.
I want the same but with a new constructor Names that inherits Array constructor.
// Contacts constructor must be the same of Array
Names
Array.prototype
I want a prototype that does not affect all arrays but only itself.. Array.prototype.test = "test" Array.prototype.t = function() {return "hello"} affects all arrays I wish to do Names.prototype.test = "test" Names.prototype.t = function() {return "hello"} where Names inherits everything else from Array prototype
– Zibri
Jul 2 at 8:44
5 Answers
5
Can't you just extend Array
?
Array
class Names extends Array {
constructor(...args) {
super(...args);
this.t = "test";
}
test() { return "hello" }
}
let z = new Names("john", "andrew")
Nope.. executing your example returns: Names [t: "test"] instead of ["john","andrew"]
– Zibri
Jul 2 at 8:38
@Zibri Sorry, my bad, I actually forgot to pass the arguments to super
– ZER0
Jul 2 at 8:55
Prototype is bind to the native type and every other element of that type will inherit prototyped properties.
If you need them for an instance only use objects.
var z = {
t : function(){
console.log("hello");
},
test : "test"
};
z.t();
console.log(z.test);
not what I asked. I want the same effect of the Array.prototype example but affecting only Names and not all arrays.
– Zibri
Jul 2 at 8:40
Here is a crude implementation:
function Names(arr) {
this.contacts = enhanceArray(arr);
}
function enhanceArray(arr) {
arr.test = 'helloProp';
arr.t = function() {
return 'helloFunc'
}
return arr;
}
let z = new Names(["john", "andrew"]);
console.log(z.contacts[0]);
console.log(z.contacts.test);
console.log(z.contacts.t());
You can create your own extended Array constructor factory, something like
(() => {
const myArr = XArray();
let a = myArr(["John", "Mary", "Michael"]);
console.log(`myArr(["John", "Mary", "Michael"]).sayHi(1): ${a.sayHi(1)}`);
console.log("demo: myArr(1, 2, 3) throws an error");
let b = myArr(1, 2, 3); // throws
// an extended array
function XArray() {
const Arr = function(arr) {
if (arr.constructor !== Array) {
throw new TypeError("Expected an array");
}
this.arr = arr;
};
Arr.prototype = {
sayHi: function (i) { return `hi ${this.arr[i]}`; }
};
return arr => new Arr(arr);
}
})();
could you check your code, it seems there is a problem when executing. ;)
– Ivan
Jul 2 at 8:46
@Ivan that's by design ;P
– KooiInc
Jul 2 at 9:04
oh sorry, I didn't pay enough attention!
– Ivan
Jul 2 at 9:22
No worries, the answer was evidently not clear enough. Edited it a bit.
– KooiInc
Jul 2 at 9:49
class Names extends Array {
constructor(...args) {
super(...args);
}
}
Names.prototype.t = 'test';
let z = new Names("john", "andrew")
z.push('Amanda')
console.log(z.t)
console.log(z)
You can easily set it at Names.prototype
Names.prototype
not what I intended to do. I want the same effect of the Array.prototype example but affecting only Names and not all arrays.
– Zibri
Jul 2 at 8:40
@Zibri: Please have a look at my updated solution.
z
can access the push method just like Array and z
can access t
as you wanted. And it doesn't pollute Array
, as you wanted– Isaac
Jul 2 at 9:54
z
z
t
Array
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
"
// Contacts constructor must be the same of Array
" this part is not clear. Do you meanNames
instances should inheritArray.prototype
as well?– Yury Tarabanko
Jul 2 at 7:09