Passing data from java file to ajax
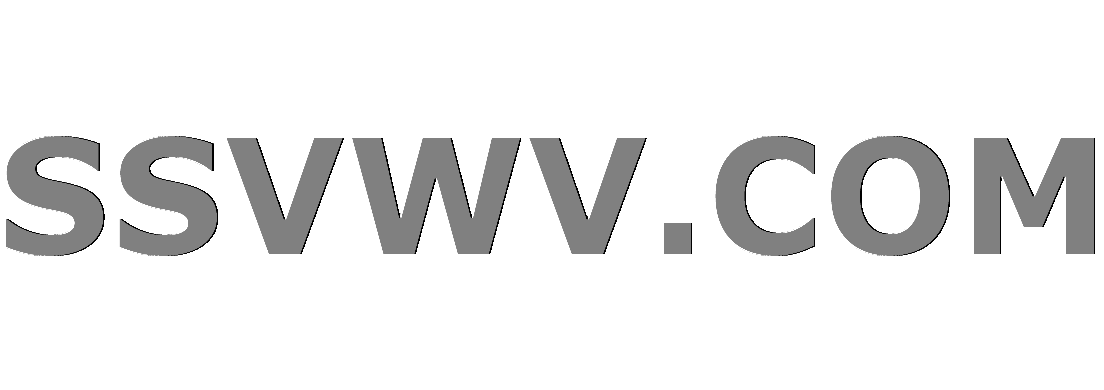
Multi tool use
Passing data from java file to ajax
I have a java class printing the progress of a process as 1%, 2%, 3%. Now I want to read this progress using AJAX requests and continuously update the progress in the webpage. I cannot convert the java file into a servlet as it is being used as an scriptlet by Jasper.
Scriptlet code is :
public void afterDetailEval() throws JRScriptletException{
count = (Integer) this.getVariableValue("count");
out.println(count);
Thread.sleep(200);
}
How can I read the data printed by java from an AJAX request?
Any help will be greatly appreciated!
what is your java class running inside? Tomcat or similar?
– William Burnham
Jul 1 at 20:40
@williamburnham yes it is running inside tomcat
– kowsikbabu
Jul 2 at 3:23
@kumasena done now
– kowsikbabu
Jul 2 at 3:25
1 Answer
1
How is your scriptlet created? The only thing that comes to mind is if you've got a class like
public class YourScriptlet extends JRDefaultScriptlet {
public void afterDetailEval() throws JRScriptletException {
// your code here
}
}
You can add a constructor and private member for another object that serves as a container for whatever you need outside of the Jasper context, like so
Some object to keep track of the count (or whatever it is you need to keep track of)
public class YourInfoObject {
private final AtomicInteger count = new AtomicInteger();
public int increment() {
return this.count.incrementAndGet();
}
public int get() {
return this.count.intValue();
}
public void set(int value) {
this.count.set(value);
}
}
Your scriptlet class with a constructor
public class YourScriptlet extends JRDefaultScriptlet {
private final YourInfoObject obj;
public YourScriptlet(YourInfoObject obj) {
this.obj = obj;
}
public void afterDetailEval() throws JRScriptletException {
// your code here
obj.set(count);
}
}
Then, from wherever else (a Servlet with a reference to your object) you can access the value.
Thank you so much for this answer!
– kowsikbabu
Jul 3 at 2:33
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
What do you think about your choice to not show the code you have that's doing the stuff you just described, while also asking for help about just these things?
– kumesana
Jul 1 at 20:07