C# webclient cannot download text from web
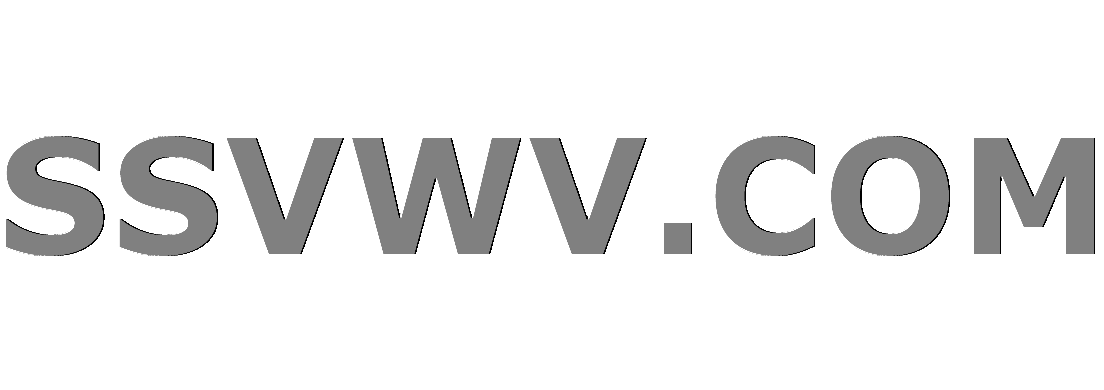
Multi tool use
C# webclient cannot download text from web
I am working on a project to get text from this website.
But when I tested it in Visual Studio, I've got some error:
Value cannot be null. Parameter name: solutionDirectory
Here is my code:
using System;
using System.Net;
public class Program
{
public static void Main()
{
WebClient client = new WebClient();
String temp = client.DownloadString("http://www.hkex.com.hk/eng/stat/dmstat/dayrpt/hsio180629.htm");
Console.Write(temp);
}
}
using
Works for me...
– TheGeneral
Jul 2 at 10:22
1 Answer
1
Please use HttpClient Which is very good Option Compare to webClient
And your problem is sounds like your project (vcxproj) file isn't setup properly.
So create New Application Simply and run this Code.
Here you find Actually Difference
Stream client = Task.Run(()=>new HttpClient().GetAsync("http://www.hkex.com.hk/eng/stat/dmstat/dayrpt/hsio180629.htm").Result.Content.ReadAsStreamAsync()).Result;
using (var fileStream = new FileStream("D://data.txt", FileMode.Create, FileAccess.Write))
{
client.CopyTo(fileStream);
}
Full Working Code:-
namespace ConsoleApp2
{
class Program
{
static void Main(string args)
{
Stream client = Task.Run(()=>new HttpClient().GetAsync("http://www.hkex.com.hk/eng/stat/dmstat/dayrpt/hsio180629.htm").Result.Content.ReadAsStreamAsync()).Result;
using (var fileStream = new FileStream("D://data.txt", FileMode.Create, FileAccess.Write))
{
client.CopyTo(fileStream);
}
}
}
}
With Await
namespace ConsoleApp2
{
class Program
{
static void Main(string args)
{
data();
Console.ReadKey();
}
public static async void data()
{
var client = await new HttpClient().GetAsync("http://www.hkex.com.hk/eng/stat/dmstat/dayrpt/hsio180629.htm");
var data= await client.Content.ReadAsStreamAsync();
using (var fileStream = new FileStream("D://data.txt", FileMode.Create, FileAccess.Write))
{
data.CopyTo(fileStream);
}
}
}
}
IIRC Task.Run is not required, since the GetAsync method is already running async when possible. And please use await, and not result. Result is blocking, await isn't.
– Mafii
Jul 2 at 11:03
whatever the methods and code are in task.run is not a blocking @Mafii
– D-john Anshani
Jul 2 at 11:07
But the call to
.Result
is, that's what I said.– Mafii
Jul 2 at 11:33
.Result
Invoking
Result
on any task that isn't complete is an anti-pattern that will eventually mess you up. Don't wait by blocking on tasks. Also, in your async example, the top-level Main
method can now be declared as async : static async Task Main(string args){}
, thereby negating the need for fire-and-forget.– spender
Jul 2 at 21:38
Result
Main
static async Task Main(string args){}
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
This is not a problem with the code you presented, which other than a missing
using
works fine.– spender
Jul 2 at 10:22