Firebase “User is missing a constructor with no arguments”
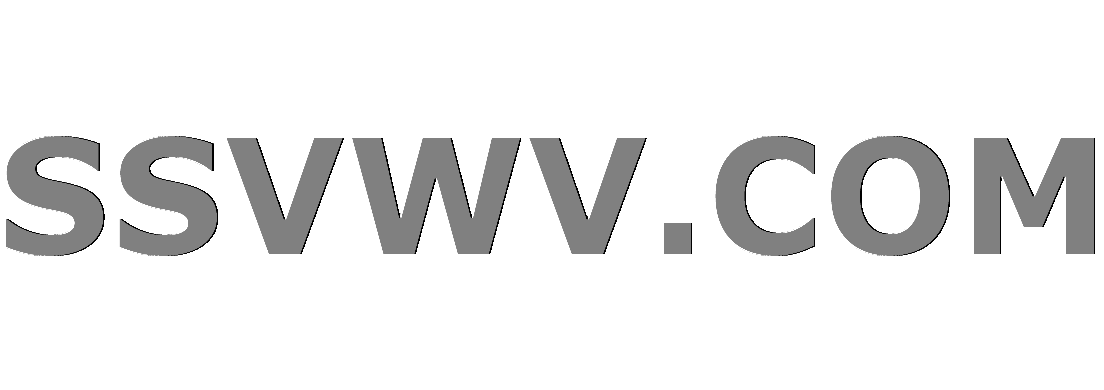
Multi tool use
Firebase “User is missing a constructor with no arguments”
I am trying to retrieve my user details from the /User/ tree i have in Firebase. I have the following User object :
public class User {
private String name;
private String email;
private FirebaseUser firebaseUser;
private String lastOnline;
private LatLng latLng;
private ArrayList<SwapLocation> locations;
public User(){ }
public String getName(){
if(firebaseUser.getDisplayName() != null && !firebaseUser.getDisplayName().equals(""))
return firebaseUser.getDisplayName();
if(name != null && !name.equals(""))
return name;
return "";
}
public void setName(String name){
this.name = name;
}
public String getEmail(){
if(firebaseUser.getEmail() != null && firebaseUser.getEmail().equals(""))
return firebaseUser.getEmail();
if(email != null && email.equals(""))
return email;
return "";
}
public void setEmail(String email){
this.email = email;
}
public void setFirebaseUser(FirebaseUser firebaseUser){
this.firebaseUser = firebaseUser;
}
public FirebaseUser getFirebaseUser(){
return firebaseUser;
}
public void setLastOnline(String lastOnline){
this.lastOnline = lastOnline;
}
public String getLastOnline(){
if(lastOnline != null)
return lastOnline;
return "";
}
public void setLatLong(LatLng latlng){
this.latLng = latlng;
}
public LatLng getLatLong(){
return this.latLng;
}
public ArrayList<SwapLocation> getLocations(){
return locations;
}
public void setLocations(ArrayList<SwapLocation> locations){
this.locations = locations;
}
}
I am trying to retrieve this object from Firebase. The object is retrieved in the dataSnapshot, although it has a problem serializing to my User object.
This is how i am retrieving the object from Firebase:
DatabaseReference ref = FirebaseDatabase.getInstance().getReference().child(users_group).child(user.getUid());
ref.addListenerForSingleValueEvent(
new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
User this_user = dataSnapshot.getValue(User.class);
}
@Override
public void onCancelled(DatabaseError databaseError) { }
});
But when trying to do 'User this_user = dataSnapshot.getValue(User.class)' i get an error saying "User is missing a constructor with no arguments"
Any help would be great, thanks
User
FirebaseUse
com.google.firebase.auth.FirebaseUser
A quick guess is that your
User
class is defined inside another class. If that is the case, mark it as static: static public class User {
. In addition, as qbix commented, I doubt FirebaseUser
meets the database requirements for serialization (would be kinda cool if it did), so you'll have to @Exclude
that.– Frank van Puffelen
Aug 6 '16 at 15:22
User
static public class User {
FirebaseUser
@Exclude
Ah damn, that sucks because i only want one User object. It seems like I need two objects, one FirebaseUser and one User for the additional properties (because the user can login without a 3rd party Auth, so those details, such as name, phone etc, need to be stored somewhere, in the 'users' tree). Or the alternative is to store the FirebaseUser in my Users object and strip it from the User object when doing calls to Firebase.. hmm what do you guys think the best solution would be?
– Nickmccomb
Aug 7 '16 at 23:25
2 Answers
2
You must be getting this error because your User class is a sub class.
Solution:
Either mark your class static:
public class SomeParentClass{
public static class User {
....
}
}
or create it in a separate file.
thanks, it worked!
– webminal.org
Sep 17 '16 at 23:44
This fixed the issue for me. The error message is tricky, since you get it whether or not your constructors include one with no arguments for whatever class you created for the database, if you define the class generically in your main activity as I originally did. The message implies an issue with the class definition, rather than the real issue of where and how it was defined.
– Androidcoder
Sep 23 '16 at 17:37
I have faced this in kotlin with firebase realtime database and solve it by this solution
For Kotlin, if you're using data class like below for firebase,
data class UserModel(
var UserName: String,
var UserType: Int
)
then you'll get an error like
com.google.firebase.database.DatabaseException: UserModel does not
define a no-argument constructor. If you are using ProGuard, make sure
these constructors are not stripped.
So for this, you need to initialize its values.
data class UserModel(
var UserName: String = "",
var UserType: Int = 0
)
While this link may answer the question, it is better to include the essential parts of the answer here and provide the link for reference. Link-only answers can become invalid if the linked page changes. - From Review
– Radosław Miernik
Jul 2 at 10:55
@RadosławMiernik done.
– KishanSolanki124
Jul 2 at 11:20
If your answer is exactly the same on the different question, then this question is a duplicate and should be closed as such.
– Luuklag
Jul 2 at 11:41
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
I can't explain why you are getting the "missing constructor" error. But I doubt Firebase will be able to serialize/deserialize your
User
class ifFirebaseUse
r is not a simple POJO you defined and instead is actuallycom.google.firebase.auth.FirebaseUser
. That class not a simple POJO and almost certainly has features that the Firebase serializer is not designed to handle.– Bob Snyder
Aug 6 '16 at 14:45