Hide an option in a spinner
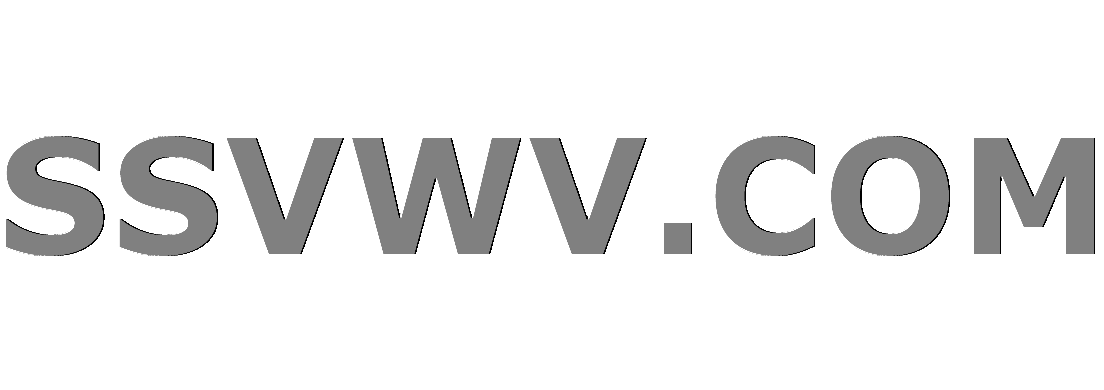
Multi tool use
Hide an option in a spinner
So I'm creating currency converting app on android I have two spinners and I'm trying to hide option from the second spinner when that option is already selected by the first spinner, like if i choose to convert from USD the second spinner USD option should disappear but I just don't have any idea how to hide an option I searched on google most question asks about hiding the whole spinner not option
package com.currencyconverter;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Spinner;
import android.widget.TextView;
import com.google.firebase.database.DatabaseReference;
import com.google.firebase.database.FirebaseDatabase;
import static com.currencyconverter.globalData.amountToBeConvert;
import static com.currencyconverter.globalData.currencies;
import static com.currencyconverter.globalData.currencyFrom;
import static com.currencyconverter.globalData.currencyTo;
public class MainActivity extends AppCompatActivity {
public Button btn;
public EditText et_AmountToBeConverted;
public Spinner sp_CurrencyFrom;
public Spinner sp_CurrencyTo;
public TextView tv_ConvertedAmount;
public TextView tv_ConvertedCurrency;
DatabaseReference databaseReference;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//Declaring XML
btn = findViewById(R.id.button);
et_AmountToBeConverted = findViewById(R.id.et_AmountToBeConverted);
sp_CurrencyFrom = findViewById(R.id.sp_CurrencyFrom);
sp_CurrencyTo = findViewById(R.id.sp_CurrencyTo);
tv_ConvertedAmount = findViewById(R.id.tv_ConvertedAmount);
tv_ConvertedCurrency = findViewById(R.id.tv_ConvertedCurrency);
// Database work
databaseReference = FirebaseDatabase.getInstance().getReference("Rates");
databaseReference.keepSynced(true);
//Inserting string to spinners
ArrayAdapter<String> adapter = new ArrayAdapter<String>(this,
android.R.layout.simple_spinner_item, currencies);
sp_CurrencyTo.setAdapter(adapter);
sp_CurrencyFrom.setAdapter(adapter);
//Spinner onseclectlistener
sp_CurrencyFrom.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() {
@Override
public void onItemSelected(AdapterView<?> adapterView, View view, int i, long l) {
currencyFrom = i;
}
@Override
public void onNothingSelected(AdapterView<?> adapterView) {
}
});
sp_CurrencyTo.getItemAtPosition((int) sp_CurrencyFrom.getSelectedItemId()).setEnabled(false);
sp_CurrencyTo.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() {
@Override
public void onItemSelected(AdapterView<?> adapterView, View view, int i, long l) {
currencyTo = i;
}
@Override
public void onNothingSelected(AdapterView<?> adapterView) {
}
});
//Convert!!
btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
//Convert XML to data
amountToBeConvert = Double.parseDouble(et_AmountToBeConverted.getText().toString());
}
});
}
}
globalData
package com.currencyconverter;
public class globalData {
public static String currencies = {"AED", "AFN", "ALL", "AMD", "ANG", "AOA", "ARS", "AUD", "AWG", "AZN", "BAM", "BBD", "BDT", "BGN", "BHD", "BIF", "BMD", "BND", "BOB", "BRL", "BSD", "BTC", "BTN", "BWP", "BYN", "BYR", "BZD", "CAD", "CDF", "CHF", "CLF", "CLP", "CNY", "COP", "CRC", "CUC", "CUP", "CVE", "CZK", "DJF", "DKK", "DOP", "DZD", "EGP", "ERN", "ETB", "EUR", "FJD", "FKP", "GBP", "GEL", "GGP", "GHS", "GIP", "GMD", "GNF", "GTQ", "GYD", "HKD", "HNL", "HRK", "HTG", "HUF", "IDR", "ILS", "IMP", "INR", "IQD", "IRR", "ISK", "JEP", "JMD", "JOD", "JPY", "KES", "KGS", "KHR", "KMF", "KPW", "KRW", "KWD", "KYD", "KZT", "LAK", "LBP", "LKR", "LRD", "LSL", "LTL", "LVL", "LYD", "MAD", "MDL", "MGA", "MKD", "MMK", "MNT", "MOP", "MRO", "MUR", "MVR", "MWK", "MXN", "MYR", "MZN", "NAD", "NGN", "NIO", "NOK", "NPR", "NZD", "OMR", "PAB", "PEN", "PGK", "PHP", "PKR", "PLN", "PYG", "QAR", "RON", "RSD", "RUB", "RWF", "SAR", "SBD", "SCR", "SDG", "SEK", "SGD", "SHP", "SLL", "SOS", "SRD", "STD", "SVC", "SYP", "SZL", "THB", "TJS", "TMT", "TND", "TOP", "TRY", "TTD", "TWD", "TZS", "UAH", "UGX", "UYU", "UZS", "VEF", "VND", "VUV", "WST", "XAF", "XAG", "XAU", "XCD", "XDR", "XOF", "XPF", "YER", "ZAR", "ZMK", "ZMW", "ZWL" };
public static double rate;
public static double amountToBeConvert;
public static int currencyFrom;
public static int currencyTo;
public static String currencies2 = {"AED", "AFN", "ALL", "AMD", "ANG", "AOA", "ARS", "AUD", "AWG", "AZN", "BAM", "BBD", "BDT", "BGN", "BHD", "BIF", "BMD", "BND", "BOB", "BRL", "BSD", "BTC", "BTN", "BWP", "BYN", "BYR", "BZD", "CAD", "CDF", "CHF", "CLF", "CLP", "CNY", "COP", "CRC", "CUC", "CUP", "CVE", "CZK", "DJF", "DKK", "DOP", "DZD", "EGP", "ERN", "ETB", "EUR", "FJD", "FKP", "GBP", "GEL", "GGP", "GHS", "GIP", "GMD", "GNF", "GTQ", "GYD", "HKD", "HNL", "HRK", "HTG", "HUF", "IDR", "ILS", "IMP", "INR", "IQD", "IRR", "ISK", "JEP", "JMD", "JOD", "JPY", "KES", "KGS", "KHR", "KMF", "KPW", "KRW", "KWD", "KYD", "KZT", "LAK", "LBP", "LKR", "LRD", "LSL", "LTL", "LVL", "LYD", "MAD", "MDL", "MGA", "MKD", "MMK", "MNT", "MOP", "MRO", "MUR", "MVR", "MWK", "MXN", "MYR", "MZN", "NAD", "NGN", "NIO", "NOK", "NPR", "NZD", "OMR", "PAB", "PEN", "PGK", "PHP", "PKR", "PLN", "PYG", "QAR", "RON", "RSD", "RUB", "RWF", "SAR", "SBD", "SCR", "SDG", "SEK", "SGD", "SHP", "SLL", "SOS", "SRD", "STD", "SVC", "SYP", "SZL", "THB", "TJS", "TMT", "TND", "TOP", "TRY", "TTD", "TWD", "TZS", "UAH", "UGX", "UYU", "UZS", "VEF", "VND", "VUV", "WST", "XAF", "XAG", "XAU", "XCD", "XDR", "XOF", "XPF", "YER", "ZAR", "ZMK", "ZMW", "ZWL" };
}
EDIT:
package com.currencyconverter;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Spinner;
import android.widget.TextView;
import com.google.firebase.database.DatabaseReference;
import com.google.firebase.database.FirebaseDatabase;
import static com.currencyconverter.globalData.amountToBeConvert;
import static com.currencyconverter.globalData.currencies;
import static com.currencyconverter.globalData.currencies2;
import static com.currencyconverter.globalData.currencyFrom;
import static com.currencyconverter.globalData.currencyTo;
public class MainActivity extends AppCompatActivity {
public Button btn;
public EditText et_AmountToBeConverted;
public Spinner sp_CurrencyFrom;
public Spinner sp_CurrencyTo;
public TextView tv_ConvertedAmount;
public TextView tv_ConvertedCurrency;
DatabaseReference databaseReference;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//Declaring XML
btn = findViewById(R.id.button);
et_AmountToBeConverted = findViewById(R.id.et_AmountToBeConverted);
sp_CurrencyFrom = findViewById(R.id.sp_CurrencyFrom);
sp_CurrencyTo = findViewById(R.id.sp_CurrencyTo);
tv_ConvertedAmount = findViewById(R.id.tv_ConvertedAmount);
tv_ConvertedCurrency = findViewById(R.id.tv_ConvertedCurrency);
// Database work
databaseReference = FirebaseDatabase.getInstance().getReference("quotes");
databaseReference.keepSynced(true);
//Inserting string to spinners
final ArrayAdapter<String> adapter = new ArrayAdapter<String>(this,
android.R.layout.simple_spinner_item, currencies);
final ArrayAdapter<String> adapter2 = new ArrayAdapter<String>(this,
android.R.layout.simple_spinner_item, currencies2);
sp_CurrencyTo.setAdapter(adapter);
sp_CurrencyFrom.setAdapter(adapter2);
//Spinner onseclectlistener
sp_CurrencyFrom.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() {
@Override
public void onItemSelected(AdapterView<?> adapterView, View view, int i, long l) {
currencyFrom = i;
}
@Override
public void onNothingSelected(AdapterView<?> adapterView) {
}
});
adapter2.remove(currencies2[sp_CurrencyFrom.getSelectedItemPosition()]);
adapter2.notifyDataSetChanged();
sp_CurrencyTo.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() {
@Override
public void onItemSelected(AdapterView<?> adapterView, View view, int i, long l) {
currencyTo = i;
}
@Override
public void onNothingSelected(AdapterView<?> adapterView) {
}
});
//Convert!!
btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
//Convert XML to data
amountToBeConvert = Double.parseDouble(et_AmountToBeConverted.getText().toString());
}
});
}
}
i.e in OnItemSelected listner of first spinner. Remove that entry from second adapter (make it member variable of class) by calling adapter.remove(String)
– Abhinav Pawar
Jul 2 at 9:54
Just use different adapters for the 2 spinners (although they're populated with the same items) and remove the item you want from the 2nd
– mTak
Jul 2 at 9:56
1 Answer
1
You can't remove items from the arrays you use for your adapters.
Declare them like this:
ArrayList<String> currencies = new ArrayList<String>(Arrays.asList("AED", "AFN", "ALL", .......));
Now you use ArrayList and you can remove items.
I edited the class, now its two adapters but remove function is crashing the app
– Kadiem Haider
Jul 2 at 10:23
Read my answer, the solution with 2 adapters is very complicated
– mTak
Jul 2 at 10:24
Sorry but I just started learning java my main question was how do I remove an option on (repopulate)
currencies
array.– Kadiem Haider
Jul 2 at 10:29
currencies
Do
currencies.remove(index);
where index
is the position of the item to be removed and then call adapter.notifyDataSetChanged();
to refresh the data in your spinner.– mTak
Jul 2 at 10:38
currencies.remove(index);
index
adapter.notifyDataSetChanged();
Can't use
currencies.remove(index);
its writing me error I should use adapter.remove(index);
and that line crashes the app.– Kadiem Haider
Jul 2 at 10:42
currencies.remove(index);
adapter.remove(index);
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
When first spinner gets selected, remove that string from second array adapter.
– Abhinav Pawar
Jul 2 at 9:51