How can I create a RAM disk programmatically?
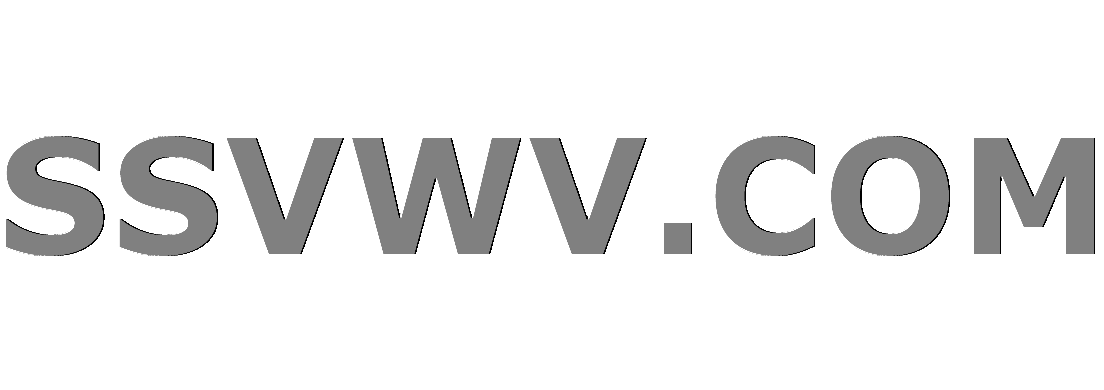
Multi tool use
How can I create a RAM disk programmatically?
I am not looking for a code that invokes a command line utility, which does the trick. I am actually interested to know the API used to create a RAM disk.
EDIT
Motivation: I have a third party library which expects a directory name in order to process the files in that directory in a certain way. I have these files zipped in an archive. I wish to extract the archive into a RAM disk and pass the third party the path to the respective directory on that RAM disk. As you can see, memory mapped files are of no use to me.
CreateRAMDisk
I edited my question.
– mark
Jan 1 '12 at 10:29
possible duplicate of Programmable RAM Disk API for .NET?
– Chris Moschini
Oct 23 '14 at 0:28
3 Answers
3
There is no API to create a RAM disk per se. You need to write your own device driver which presents a new disk to the system, and make that device driver work with allocated memory.
EDIT (after the OP's edit)
What you are trying to do would normally be done using the 'temp' directory, which is supposed to reside on the fastest disk available in the system. So, a system which already has a RAMdisk will probably have its temp environment variable already set to point to a folder on the RAMdisk, and systems without a RAMdisk would simply take the performance penalty, it is their problem.
Now, if for some reason you cannot rely on the presence of a RAMdisk on a system, AND you absolutely need the fastest performance possible, then I see no option but to install a third-party (perhaps freely downloadable, perhaps even open source) RAM disk, use it temporarily, and then uninstall it. But that's very clunky.
"write your own device driver" is synonymous to "virtually impossible" in my opinion...
– mark
Jan 1 '12 at 10:30
Yes, I think the people all over the planet who would be comfortable undertaking such a task probably fit in a room.
– Mike Nakis
Jan 1 '12 at 10:34
How long will this topic survive? Resurrection of the RAMdisk -> The SSD..
– Karel
Jan 2 '12 at 12:33
Why does the Win32 API function GetDriveType have a return value specifically for RAM disks if no other Win32 API function can create one?
– BlueMonkMN
Jan 27 '16 at 14:16
I do not know for sure, but my educated guess is that Windows obtains this bit of information from the device driver of each drive, (along with other capabilities,) so most drivers return "no", but the author of a ramdisk device driver would make their device driver return a "yes".
– Mike Nakis
Jan 27 '16 at 14:22
ImDisk is a RAM disk app that creates a virtual drive from a sector of memory, and has an API that can be called from .NET.
class RamDisk
{
public const string MountPoint = "X:";
public void createRamDisk()
{
try
{
string initializeDisk = "imdisk -a ";
string imdiskSize = "-s 1024M ";
string mountPoint = "-m "+ MountPoint + " ";
ProcessStartInfo procStartInfo = new ProcessStartInfo();
procStartInfo.UseShellExecute = false;
procStartInfo.CreateNoWindow = true;
procStartInfo.FileName = "cmd";
procStartInfo.Arguments = "/C " + initializeDisk + imdiskSize + mountPoint;
Process.Start(procStartInfo);
formatRAMDisk();
}
catch (Exception objException)
{
Console.WriteLine("There was an Error, while trying to create a ramdisk! Do you have imdisk installed?");
Console.WriteLine(objException);
}
}
/**
* since the format option with imdisk doesn't seem to work
* use the fomat X: command via cmd
*
* as I would say in german:
* "Von hinten durch die Brust ins Auge"
* **/
private void formatRAMDisk(){
string cmdFormatHDD = "format " + MountPoint + "/Q /FS:NTFS";
SecureString password = new SecureString();
password.AppendChar('0');
password.AppendChar('8');
password.AppendChar('1');
password.AppendChar('5');
ProcessStartInfo formatRAMDiskProcess = new ProcessStartInfo();
formatRAMDiskProcess.UseShellExecute = false;
formatRAMDiskProcess.CreateNoWindow = true;
formatRAMDiskProcess.RedirectStandardInput = true;
formatRAMDiskProcess.FileName = "cmd";
formatRAMDiskProcess.Verb = "runas";
formatRAMDiskProcess.UserName = "Administrator";
formatRAMDiskProcess.Password = password;
formatRAMDiskProcess.Arguments = "/C " + cmdFormatHDD;
Process process = Process.Start(formatRAMDiskProcess);
sendCMDInput(process);
}
private void sendCMDInput(Process process)
{
StreamWriter inputWriter = process.StandardInput;
inputWriter.WriteLine("J");
inputWriter.Flush();
inputWriter.WriteLine("RAMDisk for valueable data");
inputWriter.Flush();
}
public string getMountPoint()
{
return MountPoint;
}
}
Excellent tool!
– Rabih Kodeih
Jul 22 '13 at 21:55
Having tried both
IMDisk
and SoftPerfect RAM DIsk
, I prefer SoftPerfect's
.It had better performance, only reserved 0.2 MB of 100 MB allocated, versus 10 MB for IMDisk
, and didn't require the User Account Control (UAC)
prompt to mount drives. Both support a persistent option, but I did test that.– Loathing
Mar 17 '16 at 3:02
IMDisk
SoftPerfect RAM DIsk
SoftPerfect's
IMDisk
User Account Control (UAC)
you didnt mentioned the language , sO my answer is in c# :
A memory-mapped file contains the contents of a file in virtual memory. This mapping between a file and memory space enables an application, including multiple processes, to modify the file by reading and writing directly to the memory. Starting with the .NET Framework version 4, you can use managed code to access memory-mapped files in the same way that native Windows functions access memory-mapped files, as described in Managing Memory-Mapped Files in Win32 in the MSDN Library.
http://msdn.microsoft.com/en-us/library/dd997372.aspx
MemoryMappedFile MemoryMapped = MemoryMappedFile.CreateOrOpen(
new FileStream(@"C:tempMap.mp", FileMode.Create), // Any stream will do it
"MyMemMapFile", // Name
1024 * 1024, // Size in bytes
MemoryMappedFileAccess.ReadWrite); // Access type
// Create the map view and read it:
using (MemoryMappedViewAccessor FileMap = MemoryMapped.CreateViewAccessor())
{
Container NewContainer = new Container();
FileMap.Read<Container>(4, out NewContainer);
}
That is a memory mapped file. It is not a RAM disk.
– Mike Nakis
Jan 1 '12 at 9:40
@MikeNakis there are
Non-persisted memory-mapped files
and persisted memory-mapped files
msdn.microsoft.com/en-us/library/dd997372.aspx– Royi Namir
Jan 1 '12 at 9:41
Non-persisted memory-mapped files
persisted memory-mapped files
@MikeNakis
Non-persisted files are memory-mapped files that are not associated with a file on a disk.
– Royi Namir
Jan 1 '12 at 9:42
Non-persisted files are memory-mapped files that are not associated with a file on a disk.
That is correct. And memory-mapped files are not RAM disks.
– Mike Nakis
Jan 1 '12 at 9:51
I edited my question.
– mark
Jan 1 '12 at 10:29
Thank you for your interest in this question.
Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?
If you really consider RAM disk, there is no such
CreateRAMDisk
function in Windows. I think you need to look at how to create a driver for a disk, but the disk is redirected to RAM. However, if you want to simply redirect files to memory, then using memory-mapped file will do the task.– minjang
Jan 1 '12 at 9:36