How can I add files to GitLab with `python-gitlab`?
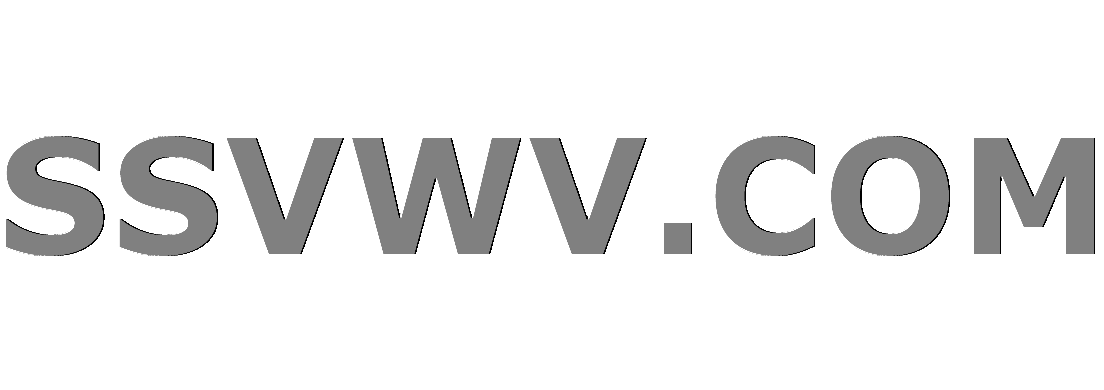
Multi tool use
How can I add files to GitLab with `python-gitlab`?
Using Django, I’d like to sync the files in the database with git repositories on my GitLab instance via python-gitlab
.
python-gitlab
Here you can find my Python code:
import gitlab
import base64
import os
from .models import Meme
from django.conf import settings
class Sync:
def sync () :
gl = gitlab.Gitlab('<GitLab URL>', private_token='xxxxxxxxxxxxxx')
for meme in Meme.objects.all():
meme_title = meme.meme_title
meme_file = str(meme.meme_file)
root = settings.MEDIA_ROOT
place = os.path.join(root, meme_file)
# Create a new project on GitLab.
project = gl.projects.create({'name': meme_title })
data = {
'branch': 'master',
'commit_message': 'Automatic commit via sync.py.',
'actions': [
{
# Binary files need to be base64 encoded
'action': 'create',
'file_path': place,
'content': base64.b64encode(open(place, "rb").read()),
'encoding': 'base64',
}
]
}
commit = project.commits.create(data)
I’m getting the following traceback:
Traceback (most recent call last):
File "/Users/keno/memeweb/lib/python3.6/site-packages/django/core/handlers/exception.py", line 35, in inner
response = get_response(request)
File "/Users/keno/memeweb/lib/python3.6/site-packages/django/core/handlers/base.py", line 128, in _get_response
response = self.process_exception_by_middleware(e, request)
File "/Users/keno/memeweb/lib/python3.6/site-packages/django/core/handlers/base.py", line 126, in _get_response
response = wrapped_callback(request, *callback_args, **callback_kwargs)
File "/Users/keno/memeweb/lib/python3.6/site-packages/django/views/decorators/csrf.py", line 54, in wrapped_view
return view_func(*args, **kwargs)
File "/Users/keno/memeweb/lib/python3.6/site-packages/django/views/generic/base.py", line 69, in view
return self.dispatch(request, *args, **kwargs)
File "/Users/keno/memeweb/lib/python3.6/site-packages/rest_framework/views.py", line 483, in dispatch
response = self.handle_exception(exc)
File "/Users/keno/memeweb/lib/python3.6/site-packages/rest_framework/views.py", line 443, in handle_exception
self.raise_uncaught_exception(exc)
File "/Users/keno/memeweb/lib/python3.6/site-packages/rest_framework/views.py", line 480, in dispatch
response = handler(request, *args, **kwargs)
File "/Users/keno/memeweb/memetree/views.py", line 42, in post
Sync.sync()
File "/Users/keno/memeweb/memetree/sync.py", line 54, in sync
commit = project.commits.create(data)
File "/Users/keno/memeweb/lib/python3.6/site-packages/gitlab/exceptions.py", line 242, in wrapped_f
return f(*args, **kwargs)
File "/Users/keno/memeweb/lib/python3.6/site-packages/gitlab/mixins.py", line 204, in create
**kwargs)
File "/Users/keno/memeweb/lib/python3.6/site-packages/gitlab/__init__.py", line 589, in http_post
post_data=post_data, files=files, **kwargs)
File "/Users/keno/memeweb/lib/python3.6/site-packages/gitlab/__init__.py", line 463, in http_request
prepped = self.session.prepare_request(req)
File "/Users/keno/memeweb/lib/python3.6/site-packages/requests/sessions.py", line 441, in prepare_request
hooks=merge_hooks(request.hooks, self.hooks),
File "/Users/keno/memeweb/lib/python3.6/site-packages/requests/models.py", line 312, in prepare
self.prepare_body(data, files, json)
File "/Users/keno/memeweb/lib/python3.6/site-packages/requests/models.py", line 462, in prepare_body
body = complexjson.dumps(json)
File "/usr/local/Cellar/python/3.6.5_1/Frameworks/Python.framework/Versions/3.6/lib/python3.6/json/__init__.py", line 231, in dumps
return _default_encoder.encode(obj)
File "/usr/local/Cellar/python/3.6.5_1/Frameworks/Python.framework/Versions/3.6/lib/python3.6/json/encoder.py", line 199, in encode
chunks = self.iterencode(o, _one_shot=True)
File "/usr/local/Cellar/python/3.6.5_1/Frameworks/Python.framework/Versions/3.6/lib/python3.6/json/encoder.py", line 257, in iterencode
return _iterencode(o, 0)
File "/usr/local/Cellar/python/3.6.5_1/Frameworks/Python.framework/Versions/3.6/lib/python3.6/json/encoder.py", line 180, in default
o.__class__.__name__)
TypeError: Object of type 'bytes' is not JSON serializable
What am I doing wrong? Why is type bytes
not fitting?
bytes
Sorry, if it is unclear, but pasted a link to my code in my question: ix.io/1fII/python.
– oioioi
Jul 2 at 10:31
Are you sure you have all this this you import, and their requirments?
– Stavros Avramidis
Jul 2 at 10:32
@StavrosAvramidis I’ve installed
Django
and python-gitlab
via pip on my virtualenv and the references to my Django model are working as well.– oioioi
Jul 2 at 10:36
Django
python-gitlab
Please don't post links to code - post the code in the question itself. I have edited your question to include the code you linked to.
– solarissmoke
Jul 2 at 11:28
1 Answer
1
The problem is that you're passing invalid data in your data
dictionary.
data
Specifically, base64.b64encode()
on Python 3 returns a bytes object, which the JSON encoder that then tries to convert this to JSON for transmission cannot handle. You can convert that bytes object to a string like so:
base64.b64encode()
'content': base64.b64encode(open(place, "rb").read()).decode("utf-8"),
Why not
.decode("ascii")
? base64-encoded text contains a rather strict subset of ASCII.– phd
Jul 2 at 14:06
.decode("ascii")
No reason - that would work too.
– solarissmoke
Jul 3 at 4:01
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
I think you need to show your code as well as the traceback
– HenryM
Jul 2 at 10:21