Images not displaying the first time in this object program in JS
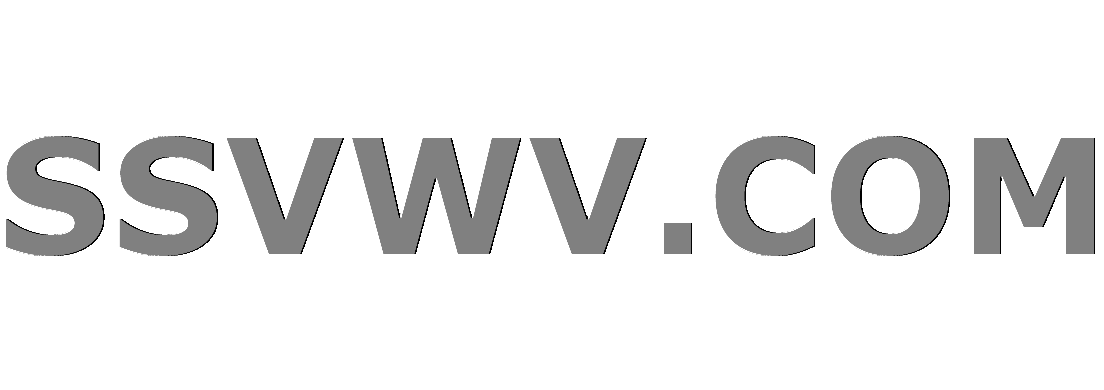
Multi tool use
Images not displaying the first time in this object program in JS
I am making a battleship game with polar coordinates. After the user chooses two points, a battleship should be drawn in the middle. My Battleship constructor looks like this:
function Battleship(size, location, source){
this.size = size;
//initializing the image
this.image = new Image();
this.image.src = source;
this.getMiddlePoint = function(){
//get midpoint of ship
...
}
this.distanceBetween = function(t1, t2){
//dist between two points
}
this.display = function(){
var point = [this.radius];
point.push(this.getMiddlePoint());
point = polarToReal(point[0], point[1] * Math.PI / 12);
//now point has canvas coordinates of midpoint
var width = this.distanceBetween(this.info[0][0], this.info[this.info.length-1][0]);
var ratio = this.image.width / width;
ctx.drawImage(this.image, point[0] - width/2, point[1] - this.image.height / ratio / 2, width, this.image.height / ratio);
//draws the image
}
}
The display
method of each ship gets called at a certain point (after the user has chosen the location). For some reason, the images do not show the first time I do this, but when I run this code at the very end:
display
for(var i = 0; i<playerMap.ships.length; i++){
playerMap.ships[i].display();
}
All ships are displayed correctly (not aligned well, but they are displayed). I think there is a problem with loading the images. I am not sure how to fix this. I tried using image.onload
but I never got that to work. I also tried something like this:
image.onload
var loadImage = function (url, ctx) {
var img = new Image();
img.src = url
img.onload = function () {
ctx.drawImage(img, 0, 0);
}
}
but the same problem kept happening. Please help me fix this problem. Here is the game in its current condition. If you place ships, nothing happens, but after you place 5 (or 10) ships, they suddenly all load.
EDIT:
I solved the problem by globally defining the images. This is still very bad practice, since I wanted this to be in the battleship object. This is my (temporary) solution:
var sub = ;
for(var i = 1; i<5; i++){
sub[i] = new Image();
sub[i].src = "/img/ships/battleship_"+i+".png";
}
BTW, your little
sub
global array has an empty first element because JS arrays are zero based indexes, e.g. first index is 0
, not 1. I assume you meant to do that to get images battleship_1 through battleship_4, but be aware of that if you loop through that array anywhere else.– Chad Moore
Jul 2 at 17:23
sub
0
Nice little game though. Good luck with your homework!
– Chad Moore
Jul 2 at 17:24
@ChadMoore Thanks for your response! I am aware of the array indexing, and it was intentional. A math teacher wanted me to make this for her algebra class haha. Yes, I'll update my code with prototypes, my bad. I realized that they are more efficient and accepted.
– Nick Solonko
Jul 2 at 18:37
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Is there a reason that you are defining all of your methods directly on the instance instead of the prototype? See developer.mozilla.org/en-US/docs/Learn/JavaScript/Objects/…. This is not why it's breaking, but just a point about OOP in JS.
– Chad Moore
Jul 2 at 17:20