Transferring Pointers from Python to dll and get modified values back
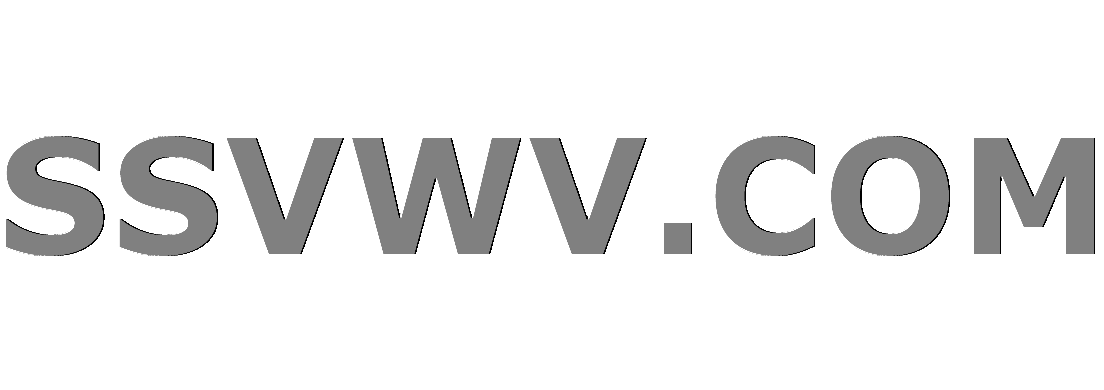
Multi tool use
Transferring Pointers from Python to dll and get modified values back
I am struggeling with the following problem, which is, I guess, not that complicated for people with more advanced C skills.
My intention is to access C++ funtions stored in an DLL from Python.
I managed to get this running for simple functions as ADD and MULT.
As the funtions I'd like to access (later) will return multiple float values I need tu use pointers.
I'd like to do the following basic steps:
-Create a variable in python,
-Transfer the pointer to the dll (ctypes --> c_pointer(...)),
-Update the value the pointer is referring to;
-go back to python and use the modified value.
My C++ funtion I used for dll creation is:
#include <stdio.h>
#include <wchar.h>
#include <iostream>
#include <vector>
#include <math.h>
#include <fstream>
using namespace::std;
extern "C" {
__declspec(dllexport)
int Kappel(double a, double *f){
*f = 25.5;
return 0;
}
} // extern "C"
My python code to call the function in the dll looks like:
import os
import os.path
import ctypes
from ctypes import pointer,c_long,c_int,c_double
lib_path=os.path.dirname(os.path.abspath(__file__))+os.path.sep+'test.dll'
lib = ctypes.CDLL(lib_path)
lib.Kappel.resttype = pointer(c_double())
p = 1.5
Cp = c_double(1.5)
Cp_ = pointer(c_double(p))
def test3(wert, zeiger):
func = lib.Kappel
Res = func(wert, zeiger)
return Res
#Output after modification
print('Returnvalue: ', test3(Cp,Cp_))
print(p)
print(Cp)
print(Cp_)
Thanks for any help
Erik
Sorry, the implied question was if somebody can identify the source of the problem in my code.
– E.Kappel
Jun 21 at 9:48
2 Answers
2
Solved the issue minutes ago.
It was as simple as supposed...
Does not work:
p = 1.5
Cp = c_double(p)
Cp_ = pointer(c_double(p))
Works:
p = 1.5
Cp = c_double(p)
Cp_ = pointer(Cp)
ctypes
has byref
, which is the preferred way to do what you want:
ctypes
byref
# Given: int Kappel(double a, double *f)
lib.Kappel.argtypes = c_double,POINTER(c_double)
lib.Kappel.restype = c_int
out = c_double() # Create instance of a C type to pass by reference.
lib.Kappel(1.5,byref(out))
print(out.value) # Retrieve the value.
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
You neglected to ask a question in your question.
– WhozCraig
Jun 21 at 8:17