Using Product CRUD setter methods in Woocommerce 3
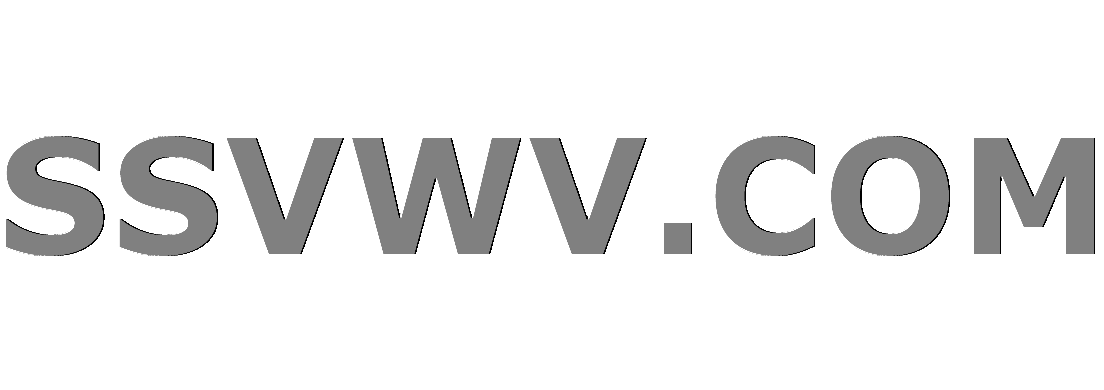
Multi tool use
Using Product CRUD setter methods in Woocommerce 3
In the code bellow, I can't set some product category and product tags:
The code is located in my functions.php file:
<?php
$product = new WC_Product;
$product->set_name("product");
$product->set_regular_price('150');
$set_cat = $product->set_category_ids( array(17) );
$set_tag = $product->set_tag_ids( [18, 19] );
$product->save();
var_dump($set_cat);//NULL
var_dump($set_tag);//NULL
The product is created with the correct name
and price
. But I get nothing for the product category and product tags:
name
price
terms:
[terms table][1]
term_taxonomy:
[term_taxonomy table][2]
Edit: I have moved this code in index.php
file and It works.
index.php
You should have a look to the answer, as there is still something wrong in your code.
– LoicTheAztec
Jul 1 at 20:42
1 Answer
1
Since Woocommerce 3, new CRUD methods are available.
But you can not use a setter method in a variables like in this extract of your code:
$set_cat = $product->set_category_ids( array(17) );
$set_tag = $product->set_tag_ids( [18, 19] );
Instead, it should be only:
$product->set_category_ids( array(17) );
$product->set_tag_ids( [18, 19] );
$product->save();
Then just after you will use getter methods to read the saved data and display it:
$get_cats = $product->get_category_ids();
$get_tags = $product->get_tag_ids();
var_dump($get_cats); // NOW OK
var_dump($get_tags); // NOW OK
For function.php
file you should embed you code in a function like:
function.php
function my_custom_function_code(){
// Get a new empty WC_Product instance object
$product = new WC_Product;
# Setter methods (set the data)
$product->set_name("product");
$product->set_regular_price('150');
$product->set_category_ids( array(17) );
$product->set_tag_ids( [18, 19] );
# Save the data
$product->save(); // Always at the end to save the new data
# Getter methods (Read the data)
$get_cats = $product->get_category_ids();
$get_tags = $product->get_tag_ids();
# Display some raw data
var_dump($get_cats); // NOW OK
var_dump($get_tags); // NOW OK
}
Then you can use it anywhere else (like in your index.php file) simply with:
my_custom_function_code();
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Could you add a bit more clarification to this question, what exactly are you trying to do and what aspect of it is not working, have you tried anything else to address the issue?
– David Rogers
Jul 1 at 16:27