Where Can I Download Rest API [closed]
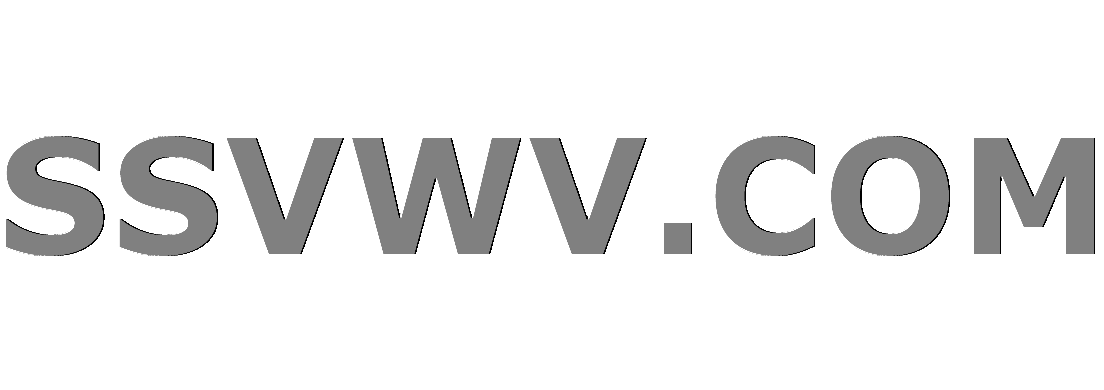
Multi tool use
Where Can I Download Rest API [closed]
This might be a stupid question, but a school project I am working on involves us using Java and the Rest API. I can't seem to find where to download the API.
Shouldn't it just be a jar file that I add to my build path? I can't seem to find a download anywhere which makes me think I am incredibly confused.
Any help would be greatly appreciated. I am sorry if this is a duplicated question, but every single thread I have found is just about downloading a file USING the rest api.
Thanks.
Please clarify your specific problem or add additional details to highlight exactly what you need. As it's currently written, it’s hard to tell exactly what you're asking. See the How to Ask page for help clarifying this question. If this question can be reworded to fit the rules in the help center, please edit the question.
Sounds like your project may involve building and using a rest api, look into something like jersey or spring frameworks. then start with a simple hello world tutorial, spring.io/guides/gs/rest-service
– davedwards
Jul 1 at 21:09
2 Answers
2
A rest api is something you build yourself. Basically you build a webservice to deliver data to some other application/frontend etc.
To get started with REST in Java I would recommend using a simple Framework like Spring (see: https://spring.io/guides/gs/spring-boot/ )
Then what you basically do is the following.
Please do note this is an example using jax.rs, another Java framework, which I found harder for the beginning. Neverthless in SpringBoot you will basically be doing the same.
@GET
@Path("bills")
public ResultSetIterator getBills(
@Context HttpServletRequest request,
@QueryParam("id") @DefaultValue("-1") long id) {
Connection con = null;
ResultSet rs = null;
PreparedStatement stmt = null;
try {
con = this.sqlAdapter.getConnection();
String sql = "SELECT * FROM mytable WHERE id= ? ;
stmt = con.prepareStatement(sql);
stmt.setLong(1,id);
rs = stmt.executeQuery();
return new ResultSetIterator(rs, con);
} catch (Exception e) {
}
return null;
}
You "hook" your method to a path of your webserver
@Path("bills")
And define what kind of request you expect.
@GET
Depending on your Parameters
@QueryParam("id") @DefaultValue("-1") long id)
You then have your method return different data.
Do some reading on RESTful Webservices in general and then revisit implementing one in Java, the language really does not matter here.
A RESTful API is an application program interface (API) that uses HTTP
requests to GET, PUT, POST and DELETE data.
You don't download an API but you should look for a framework to help you build Restful Api
.
Restful Api
Check How I explained REST to my wife
and What exactly is RESTful programming?
then check Getting Started · Building a RESTful Web Service
SVI,gn LBPvylu hPBjt,C,17d0VNcN7IvA3cED JXA,OOB gcIDOowGienz SY tDIy cRDfrVsf4P 7pFQKUl J6uu8ceq 9Rzw9BfKNuBlV0c,D
You can use Jersey Framework. Add your J2EE Web application to this framework .jersey.github.io
– anilkay
Jul 1 at 21:08