arrangement of names with same starting alphabets
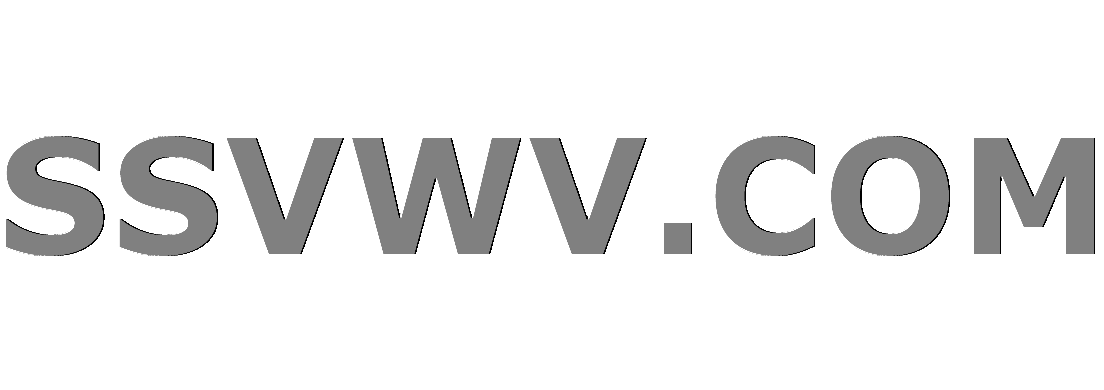
Multi tool use
arrangement of names with same starting alphabets
public class solution{
public static void main(String args){
String priya={"priya","nandhni","nithesh","varan","rekha","sri"};
System.out.println(priya);
int n=priya.length;
for(int i=0,j=i+1;i<n;i++){
if(priya[i].compareTo(priya[j])>0)
{
String temp=priya[i];
priya[i]=priya[j];
priya[j]=temp;
}
}
for(int i=0;i<n;i++){
System.out.println(priya[i]);
}
}
}
The output for ascending order comes as
nandhni
varan
nithesh
priya
rekha
sri.
what could the mistake here?
Hint: most sort algorithms must involve some sort of nested loop. Since you only have one loop, the most you can do is one swap per array element (in your case, per adjacent elements). Start with something simple like a bubble sort, and attempt to hand execute it to understand why one loop isn't enough.
– markspace
Jul 1 at 17:37
you want to sort this array (by any way ) you want to make the algorythm of sort ? if you want the algorythme wich kind of algorythme you want?
– Arnault Le Prévost-Corvellec
Jul 1 at 19:32
2 Answers
2
Try this one. Nested loop so that not only adjacent elements are compared and inner loop terminates at last element.
for (int i = 0; i < n-1; i++) {
for (int j = i + 1; j < n; j++) {
if (priya[i].compareTo(priya[j]) > 0) {
String temp = priya[i];
priya[i] = priya[j];
priya[j] = temp;
}
}
}
The sorting logic is wrong, You are just doing one pass of element comparison
Correct one as below:
for (int i = 0; i < n; i++) {
for (int j = i + 1; j < n; j++) {
if (priya[i].compareTo(priya[j]) > 0) {
String temp = priya[i];
priya[i] = priya[j];
priya[j] = temp;
}
}
}
stiill not working :(. it gets printed as nandhni nithesh priya varan rekha sri
– priya priya
Jul 1 at 18:12
but nandhni nithesh priya varan rekha sri is the correct order?
– jos.mathew123
Jul 1 at 18:20
No, varan is out of order and should come at the end of the list.
– markspace
Jul 1 at 19:28
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Your code is not a complete sort. You only consider directly adjacent pairs of names once.
– Elliott Frisch
Jul 1 at 17:28