Replace number in word
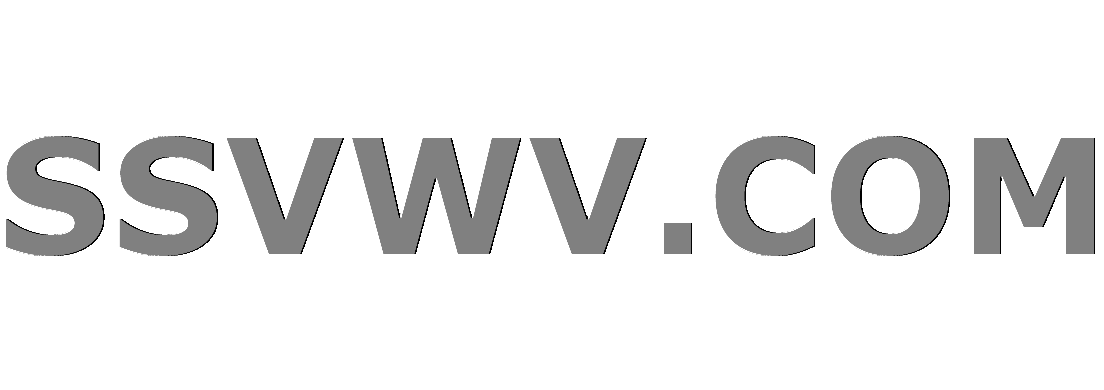
Multi tool use
Replace number in word
How is it possible to replace every 1
with one
, every 2
with two
, every 3
with three
...
from an Input?
1
one
2
two
3
three
My Code:
import javax.swing.JOptionPane;
public class Main {
public static void main(String args) {
String Input = JOptionPane.showInputDialog("Text:");
String Output;
//replace
Output = Input.replaceAll("1", "one");
Output = Input.replaceAll("2", "two");
//Output
System.out.println(Output);
}
}
It just work with one replace-item.
1
one
123
No. It schut work like that:
– Marek
Jul 1 at 15:11
Input : 1 2 Output: one two
– Marek
Jul 1 at 15:11
Please don't put additional information into comments, instead update your question.
– GhostCat
Jul 1 at 15:12
Please stick to naming conventions. Variable names always camelCase. So
input
and output
.– Zabuza
Jul 1 at 18:06
input
output
2 Answers
2
You need call replaceAll
on OutPut
for the second time:
replaceAll
OutPut
Output = Input.replaceAll("1", "one");
Output = Output.replaceAll("2", "two");
or just call replaceAll
fluently:
replaceAll
Output = Input.replaceAll("1", "one").replaceAll("2", "two");
Thank you very much!
– Marek
Jul 1 at 15:18
Your code is setting Output
twice using Input
as the source string. Therefore, calling Output = Input.replaceAll("2", "two);
completely negates the first time you called it.
Output
Input
Output = Input.replaceAll("2", "two);
You could replace that with this instead:
Output = Input.replaceAll("1", "one");
Output = Output.replaceAll("2", "two");
But that would be a bit excessive and become quite cumbersome if you want to define a lot of replacements.
HashMap
Using HashMap<Character, String>
allows you to store the single-character "key," or the value you want to replace, and its replacement string.
HashMap<Character, String>
Then it is just a matter of reading each character of the input string and determining when the HashMap
has defined a replacement for it.
HashMap
import java.util.HashMap;
public class Main {
private static HashMap<Character, String> replacementMap = new HashMap<>();
public static void main(String args) {
// Build the replacement strings
replacementMap.put('1', "one");
replacementMap.put('2', "two");
replacementMap.put('3', "three");
replacementMap.put('4', "four");
replacementMap.put('5', "five");
replacementMap.put('6', "six");
replacementMap.put('7', "seven");
replacementMap.put('8', "eight");
replacementMap.put('9', "nine");
replacementMap.put('0', "zero");
String input = "This is 1 very long string. It has 3 sentences and 121 characters. Exactly 0 people will verify that count.";
StringBuilder output = new StringBuilder();
for (char c : input.toCharArray()) {
// This character has a replacement defined in the map
if (replacementMap.containsKey(c)) {
// Add the replacement string to the output
output.append(replacementMap.get(c));
} else {
// No replacement found, just add this character to the output
output.append(c);
}
}
System.out.println(output.toString());
}
}
Output:
This is one very long string. It has three sentences and onetwotwo characters. Exactly zero people will verify this count.
Limitations:
First of all, this implementation depends on your desired functionality and scope. Since there are an infinite number of possible numbers, this would not account for that.
Also, this looks for a single character to replace. If you wanted to expand this to replace "10" with "ten," for example, you would need to use HashMap<String, String>
instead.
HashMap<String, String>
Unfortunately, your original question does provide enough context in order to suggest the best way for you.
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
What is the context for the replacements? Do you want to really replace every digit
1
withone
, even if the former appears inside a large number, e.g.123
?– Tim Biegeleisen
Jul 1 at 15:09