angular 5/6: ERROR Error: mat-form-field must contain a MatFormFieldControl
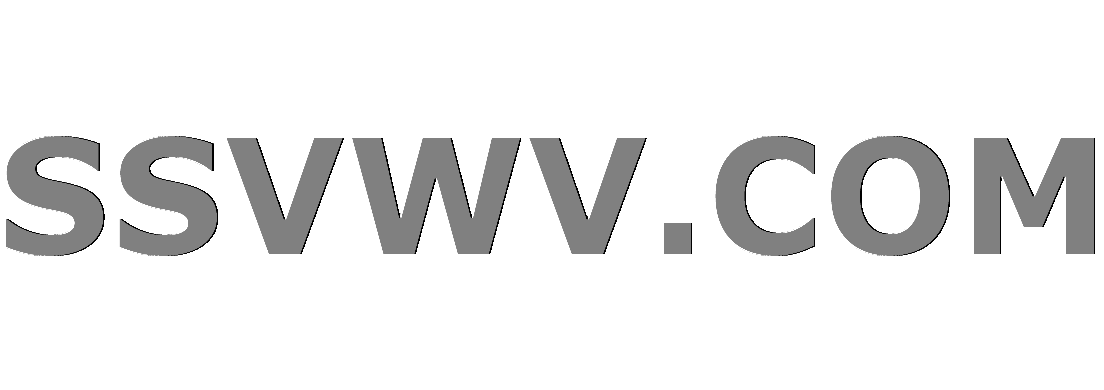
Multi tool use
angular 5/6: ERROR Error: mat-form-field must contain a MatFormFieldControl
I'm trying to open an OpenDialog window from a table list and I'm receiving this error:
ERROR Error: mat-form-field must contain a MatFormFieldControl.
here is the code of the HTML openDialog:
<h2 mat-dialog-title>Edit Order</h2>
<mat-dialog-content >
<mat-form-field>
<mat-card-content *ngIf="order.orderDate != null" [(ngModel)]="order.orderDate" name="orderDate" ngDefaultControl ></mat-card-content>
</mat-form-field>
<mat-form-field>
<mat-card-content [(ngModel)]="order.destAddress" name="destAddress" ngDefaultControl></mat-card-content>
</mat-form-field>
<mat-form-field>
<mat-card-content [(ngModel)]="order.deliveryType" name="deliveryType" ngDefaultControl></mat-card-content>
</mat-form-field>
<mat-form-field>
<mat-card-content [(ngModel)]="order.price" name="price" ngDefaultControl ></mat-card-content>
</mat-form-field>
<mat-form-field>
<mat-card-content [(ngModel)]="order.status" name="status" ngDefaultControl></mat-card-content>
</mat-form-field>
</mat-dialog-content>
<mat-dialog-actions>
<button class="mat-raised-button"(click)="close()">Close</button>
</mat-dialog-actions>
here is the code of the Dialog Component
import { Component, OnInit, Inject } from '@angular/core';
import { MatDialogRef, MAT_DIALOG_DATA} from '@angular/material';
import { Order } from '../Order';
import { OrdersService} from '../orders.service';
export interface DialogData {
orderId: number;
customerId: number;
motoboyId: number;
orderDate: Date;
localAddress: string;
latitudeOriginAddress: number;
longitudeOriginAddress: number;
destAddress:string;
latitudeDestAddress:number;
longitudeDestAddress: number;
price: number;
collectDate: Date;
deliveryDate: Date;
contactDestination: string;
phoneDestination:string;
phoneNumber: string;
deliveryType: string;
status: string;
active: boolean;
}
@Component({
selector: 'app-order-screen',
templateUrl: './order-screen.component.html',
styleUrls: ['./order-screen.component.css']
})
export class OrderScreenComponent implements OnInit {
showButton : boolean = true;
order = new Order();
constructor(private orderService: OrdersService, public dialogRef: MatDialogRef<OrderScreenComponent>, @Inject(MAT_DIALOG_DATA) public data: DialogData) {
console.log(data);
this.order = data;
}
ngOnInit() {
}
close() {
this.dialogRef.close();
}
}
here is the code of the HTML from where the OpenDialog has to be open:
<div>
<mat-form-field>
<input matInput (keyup)="applyFilter($event.target.value)" placeholder="Filter">
</mat-form-field>
<button (click)="refresh()">refresh</button>
<mat-table [dataSource]="dataSource">
<ng-container matColumnDef="orderId">
<mat-header-cell *matHeaderCellDef> Order Id </mat-header-cell>
<mat-cell *matCellDef="let order"> {{order.orderId}} </mat-cell>
</ng-container>
<ng-container matColumnDef="customerId">
<mat-header-cell *matHeaderCellDef> Customer </mat-header-cell>
<mat-cell *matCellDef="let order"> {{order.customer.firstName}} </mat-cell>
</ng-container>
<ng-container matColumnDef="motoboyId">
<mat-header-cell *matHeaderCellDef> Moto Boy </mat-header-cell>
<mat-cell *matCellDef="let order"> {{order.motoboy.firstName}} </mat-cell>
</ng-container>
<ng-container matColumnDef="localAddress">
<mat-header-cell *matHeaderCellDef> Local Address </mat-header-cell>
<mat-cell *matCellDef="let order"> {{order.localAddress}} </mat-cell>
</ng-container>
<ng-container matColumnDef="destAddress">
<mat-header-cell *matHeaderCellDef> Destenation Address </mat-header-cell>
<mat-cell *matCellDef="let order"> {{order.destAddress}} </mat-cell>
</ng-container>
<ng-container matColumnDef="price">
<mat-header-cell *matHeaderCellDef> Price </mat-header-cell>
<mat-cell *matCellDef="let order"> {{order.price}} </mat-cell>
</ng-container>
<ng-container matColumnDef="orderDate">
<mat-header-cell *matHeaderCellDef> Order Date </mat-header-cell>
<mat-cell *matCellDef="let order"> {{order.orderDate}} </mat-cell>
</ng-container>
<ng-container matColumnDef="active">
<mat-header-cell *matHeaderCellDef> isActive </mat-header-cell>
<mat-cell *matCellDef="let order">
<button *ngIf="order.motoboy === null" (click)="handleAsignToOrder(order)" >assign</button>
</mat-cell>
</ng-container>
<ng-container matColumnDef="actions">
<mat-header-cell *matHeaderCellDef> Actions </mat-header-cell>>
<mat-cell *matCellDef="let order">
<button matTooltip="Edit Order" mat-raised-button (click)="openDialog(order.orderId)" >Edit</button>
</mat-cell>>
</ng-container>
<mat-header-row *matHeaderRowDef="displayedColumns"></mat-header-row>
<mat-row *matRowDef="let row; columns: displayedColumns;"></mat-row>
</mat-table>
</div>
and here is the code of the component belongs to the HTML.
import { Component, OnInit, Inject } from '@angular/core';
import { MatDialog, MatDialogRef, MatDialogConfig, MAT_DIALOG_DATA } from '@angular/material';
import { Order } from '../Order'
import { Observable } from 'rxjs';
import { OrdersService } from '../orders.service'
import { MatTableDataSource } from '@angular/material';
import { MotoBoy } from '../MotoBoy';
import { MotoService } from '../moto.service';
import { OrderScreenComponent } from '../order-screen/order-screen.component';
@Component({
selector: 'app-table',
templateUrl: './table.component.html',
styleUrls: ['./table.component.css']
})
export class TableComponent implements OnInit {
order: Order;
currentMotoBoy: MotoBoy = new MotoBoy();
orders: Array<Order> = new Array<Order>();
dataSource = new MatTableDataSource(this.orders);
displayedColumns = ['orderId', 'customerId', 'motoboyId', 'localAddress', 'destAddress', 'price', 'orderDate', 'active', 'actions'];
constructor(private ordersService: OrdersService, private motoService: MotoService, public dialog: MatDialog) {
}
ngOnInit() {
// this.dataSource.data === this.order used this way to filter
this.dataSource.data = this.ordersService.allOrders;
this.ordersService.getAllOrders();
this.ordersService.allOrdersObservable.subscribe((data) => {
this.dataSource.data = data;
console.log(this.dataSource.data)
})
this.motoService.singleMotoObservable.subscribe((data) => {
this.currentMotoBoy = data;
console.log(this.currentMotoBoy)
})
}
openDialog(order_id) {
let order = this.ordersService.findOrder(order_id)
const dialogConfig = new MatDialogConfig();
dialogConfig.disableClose = true;
dialogConfig.autoFocus = true;
dialogConfig.data = order
console.log(order);
this.dialog.open(OrderScreenComponent, dialogConfig);
}
}
I will enjoy to you will help me.
Thank you
2 Answers
2
You have to set matInput
directive on an input
html element inside your mat-form-field
element.
matInput
input
mat-form-field
See https://material.angular.io/components/form-field/overview for more info.
Solution is that you need to include matInput directive with mat-form-feild or just use simple tag
<h2 mat-dialog-title>Edit Order</h2>
<mat-dialog-content >
<div>
<mat-card-content *ngIf="order.orderDate != null" [(ngModel)]="order.orderDate" name="orderDate" ngDefaultControl ></mat-card-content>
<div>
<mat-card-content [(ngModel)]="order.destAddress" name="destAddress" ngDefaultControl></mat-card-content>
</div>
<div>
<mat-card-content [(ngModel)]="order.deliveryType" name="deliveryType" ngDefaultControl></mat-card-content>
</div>
<div>
<mat-card-content [(ngModel)]="order.price" name="price" ngDefaultControl ></mat-card-content>
</div>
<div>
<mat-card-content [(ngModel)]="order.status" name="status" ngDefaultControl></mat-card-content>
</div>
</mat-dialog-content>
<mat-dialog-actions>
<button class="mat-raised-button"(click)="close()">Close</button>
</mat-dialog-actions>
simple tag means remove <mat-form-field></mat-form-field> instead of that use <div></div>
– UnluckyAj
Jul 2 at 4:47
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
What do you mean when you you said to just use simple tag? could you give an example? thank
– user2422556
Jul 1 at 20:30