Get Javascript object array from table data
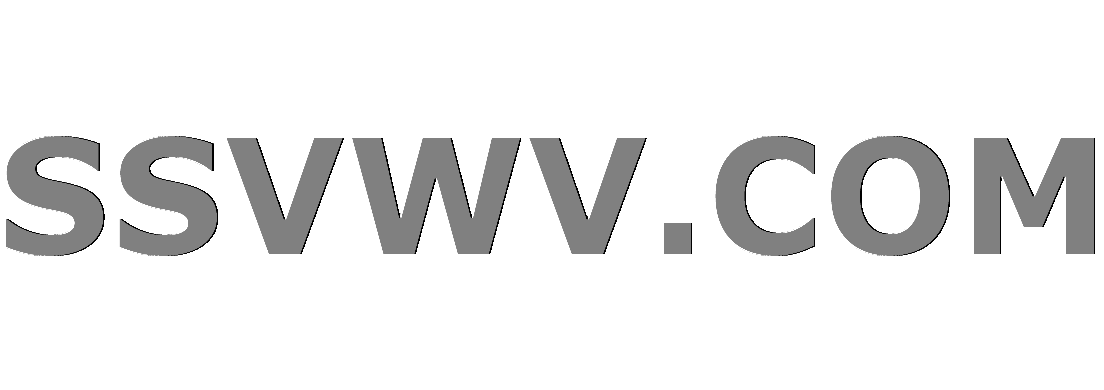
Multi tool use
Get Javascript object array from table data
I am likely new at javascript, i want to extract data from table in json object format
I have a table look like this
<table>
<thead>
<tr>
<th class="active">Bolumn</th>
<th class="active">Column</th>
<th class="active">Dolumn</th>
<th>Molumn</th>
</tr>
</thead>
<tbody>
<tr>
<td class="active">Bolumn Data</td>
<td class="active">Column Data</td>
<td class="active">Dolumn Data</td>
<td>Molumn Data</td>
</tr>
<tr>
<td class="active">Bolumn Data 1</td>
<td class="active">Column Data 1</td>
<td class="active">Dolumn Data 1</td>
<td>Molumn Data 1</td>
</tr>
<tr>
<td class="active">Bolumn Data 2</td>
<td class="active">Column Data 2</td>
<td class="active">Dolumn Data 2</td>
<td>Molumn Data 2</td>
</tr>
</tbody>
</table>
In table some of have active class and i want only this active class data
So I want json formated look like this and i want in jquery method
[{"Bolumn":"Bolumn Data","Column":"Column Data","Dolumn":"Dolumn Data"},
{"Bolumn":"Bolumn Data 1","Column":"Column Data 1","Dolumn":"Dolumn Data 1"},
{"Bolumn":"Bolumn Data 2","Column":"Column Data 2","Dolumn":"Dolumn Data 2"}]
Thanks in Advance
Updated:
I've tried like this code but i don't how to achieve this
var array = ;
$('tr').each(function (i) {
$(this).find('td').each(function (i) {
if ($(this).hasClass('active')) {
array.push($(this).text());
}
});
});
Good or not, you need to make an effort of your own, so provide what you have so far using jQuery
– LGSon
Jul 1 at 17:35
I've tried lot way but success @TylerRoper
– Tawhidul Islam
Jul 1 at 17:37
Show us what looks like your best try in a Minimal, Complete, and Verifiable example - click the
<>
- you need to run over $("th.active")
and $("td.active")
– mplungjan
Jul 1 at 17:38
<>
$("th.active")
$("td.active")
Thanks for adding the code to your answer. It makes it a lot easier to provide a useable answer.
– Mark Meyer
Jul 1 at 18:09
2 Answers
2
Your code is quite close to working. It needs a few things however to get your desired results. First, since you want objects, you need the keys, which are found in the header. You can make an array of these the same way you are doing for your data:
var headers =
$('tr th').each(function (i) {
headers.push($(this).text())
})
Now you can reference the headers by index in your loop and assign values to the keys as you go:
// find headers
var headers =
$('tr th').each(function(i) {
headers.push($(this).text())
})
// result array
var array = ;
$('tr').each(function(i) {
// declare object variable but dont set it's value
// unless there are objects to find
var rowObj
$(this).find('td').each(function(i) {
if (!rowObj) rowObj = {}
if ($(this).hasClass('active')) {
// use the header we found earlier
rowObj[headers[i]] = $(this).text()
}
});
// if we found active objects, rowObje will be defined
if (rowObj) array.push(rowObj)
});
console.log(array)
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<table>
<thead>
<tr>
<th class="active">Bolumn</th>
<th class="active">Column</th>
<th class="active">Dolumn</th>
<th>Molumn</th>
</tr>
</thead>
<tbody>
<tr>
<td class="active">Bolumn Data</td>
<td class="active">Column Data</td>
<td class="active">Dolumn Data</td>
<td>Molumn Data</td>
</tr>
<tr>
<td class="active">Bolumn Data 1</td>
<td class="active">Column Data 1</td>
<td class="active">Dolumn Data 1</td>
<td>Molumn Data 1</td>
</tr>
<tr>
<td class="active">Bolumn Data 2</td>
<td class="active">Column Data 2</td>
<td class="active">Dolumn Data 2</td>
<td>Molumn Data 2</td>
</tr>
</tbody>
</table>
As an alternative to a jQuery solution, here is mine with vanilla js and ES6 that utilizes Array.prototype.map
and Array.prototype.reduce
.
Array.prototype.map
Array.prototype.reduce
Learning those would be useful in the future and will help you write more declarative code.
// 1) extract headers and rows in separate arrays with
// respective `isActive` flag for each item in both
var [headers, ...rows] = [...document.querySelectorAll('tr')].map(
tr => [...tr.querySelectorAll('*')].map(n => ({
name: n.innerText,
isActive: n.classList.contains('active')
})));
// 2) iterate over the `rows` and dynamically aggregate the output object
// by matching headers and rows based on their indexes:
var result = rows.map(row => {
return headers.reduce((obj, h, j) => {
if (h.isActive && row[j].isActive) {
obj[h.name] = row[j].name;
}
return obj;
}, {});
});
console.log(result);
<table>
<thead>
<tr>
<th class="active">Bolumn</th>
<th class="active">Column</th>
<th class="active">Dolumn</th>
<th>Molumn</th>
</tr>
</thead>
<tbody>
<tr>
<td class="active">Bolumn Data</td>
<td class="active">Column Data</td>
<td class="active">Dolumn Data</td>
<td>Molumn Data</td>
</tr>
<tr>
<td class="active">Bolumn Data 1</td>
<td class="active">Column Data 1</td>
<td class="active">Dolumn Data 1</td>
<td>Molumn Data 1</td>
</tr>
<tr>
<td class="active">Bolumn Data 2</td>
<td class="active">Column Data 2</td>
<td class="active">Dolumn Data 2</td>
<td>Molumn Data 2</td>
</tr>
</tbody>
</table>
I would be more specific:
...tr.querySelectorAll('td, th')
– mplungjan
Jul 2 at 16:37
...tr.querySelectorAll('td, th')
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
If you find that your implied question is "Can someone please do this for me?", then it's likely not yet at a stage where it should be asked on StackOverflow. Please take a few minutes to familiarize with How To Ask, and make an attempt before requesting help.
– Tyler Roper
Jul 1 at 17:35