Java8 enum avoid multiple if else
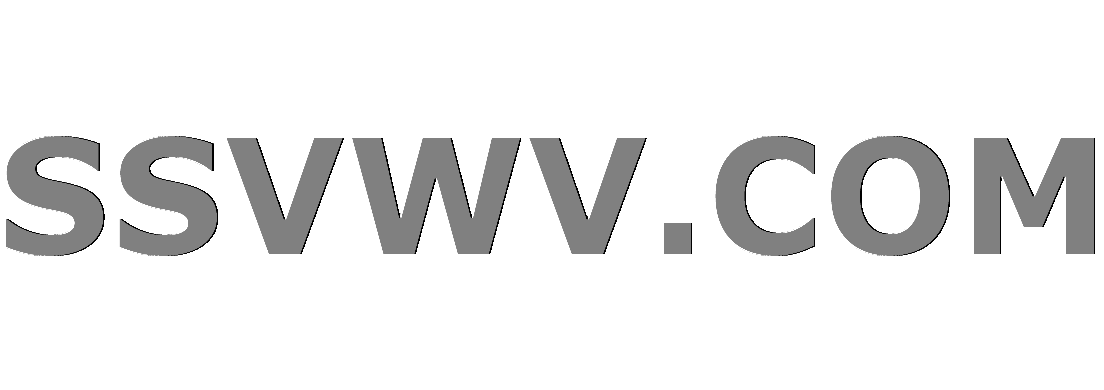
Multi tool use
Java8 enum avoid multiple if else
In java 8 is there any option to avoid multiple if else check with enum value and to execute particular operation. I dont like to use some thing like below example ?
if enum equals A
PRINT A
else if enum equals B
PRINT B
else if enum equlas C
PRINT C
You do not need to check the equality if the operation is always the same
– Yassin Hajaj
Jul 1 at 18:06
2 Answers
2
Define enum with abstract method and provide it's implementation with values.
enum MyEnum{
A{
@Override
public void doSomething() {
// Logic goes here
}
},
B{
@Override
public void doSomething() {
// Logic goes here
}
}
abstract public void doSomething ();
}
Now, you can directly call the required method without an if.
MyEnum.B.doSomething();
A method receives a enum value and based on the enum value different actions will needs to be executed.So without if condition ,will the code snippet above can be implemented?
– java_01
Jul 1 at 18:10
Yes, you just need to call doSomething method on the enum value inside the method which receives enum.
– Vaibhav Raj
Jul 1 at 18:12
What you are looking for is switch
statements. And not just in Java 8, you can switch on enums
in all previous versions of Java. Consider the following code:
switch
enums
public class Dummy {
enum MyENUM {
A,
B,
C
}
public static void main(final String args) {
MyENUM myENUM = MyENUM.A;
switch (myENUM) {
case A:
System.out.println(MyENUM.A);
break;
case B:
System.out.println(MyENUM.B);
break;
case C:
System.out.println(MyENUM.C);
break;
}
}
}
If you don't want to use switch
statements, This page provides a various alternative to switch
statements.
switch
switch
One of the ways to replace switch
is to create a Map. Consider below example:
switch
public static void main(final String args) {
Map<MyENUM,Runnable> map = new HashMap<>();
map.put(MyENUM.A,() -> System.out.println(MyENUM.A));
map.put(MyENUM.B,() -> System.out.println(MyENUM.B));
map.put(MyENUM.C,() -> System.out.println(MyENUM.C));
MyENUM myENUM = MyENUM.A;
map.get(myENUM).run();
}
Produces following result:
A
Thanks.Can this be simplified without using switch statement in java8.. ?
– java_01
Jul 1 at 17:51
@java_01 Updated the answer
– Pankaj Singhal
Jul 1 at 18:04
You can replace the entire
switch
statement with System.out.println(myEnum);
…– Holger
Jul 2 at 7:28
switch
System.out.println(myEnum);
@Holger The purpose of OP's Question is NOT printing the enum. It's more of finding an alternative to
if-else
. Me, writing System.out.println(MyENUM.A)
is basically showing some example of statement execution.– Pankaj Singhal
Jul 2 at 8:51
if-else
System.out.println(MyENUM.A)
@PankajSinghal there is no indicator that the OP’s actual use case isn’t as superfluous as the given example which isn’t even valid Java syntax. Maybe the information that Java supports non-constant parameters which allow to avoid code duplication is valuable to the OP…
– Holger
Jul 2 at 9:03
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Is it possible you're looking for switch statements?
– Silvio Mayolo
Jul 1 at 17:37