How does Unity distinguish between Android and iOS
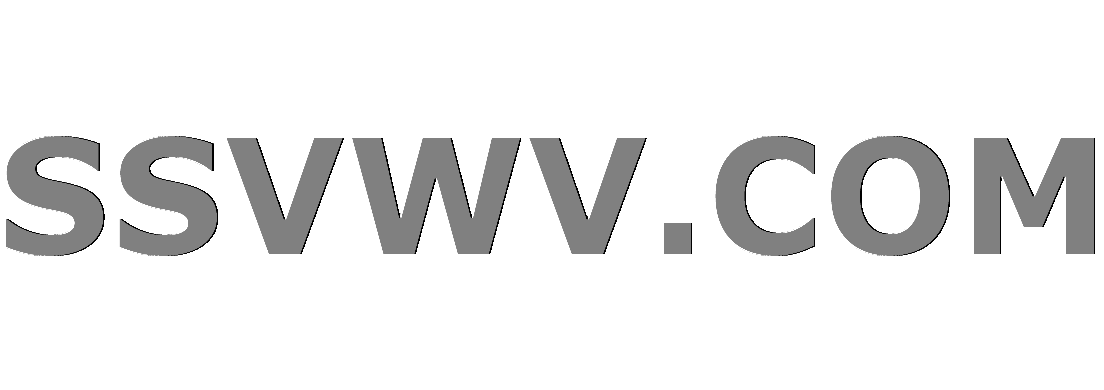
Multi tool use
How does Unity distinguish between Android and iOS
Let's say we have make a mobile game based on accelerometer. We're using Input.accelerometer class.
using UnityEngine;
public class ExampleClass : MonoBehaviour {
public float speed = 10.0F;
void Update() {
Vector3 dir = Vector3.zero;
dir.x = -Input.acceleration.y;
dir.z = Input.acceleration.x;
if (dir.sqrMagnitude > 1)
dir.Normalize();
dir *= Time.deltaTime;
transform.Translate(dir * speed);
}
}
This code works on Andoid and on iOS. Im wondering, how does Unity know if we're running the game on Android or iOS? I have checked UnityEngine.dll file and I did not find any if-else statement between the operating systems.
Also, I believe most developers would use a preprocess command to prevent unnecessary code from being compiled thiings like #if #elif #else. If you are compiling a .so or even a .dll and your #if condition isn't true, then the code in that body wouldn't be included with the finished object.
– Eddge
Jul 1 at 16:34
The target platform is decided at build time, you won't find anything in the library files.
– UnholySheep
Jul 1 at 16:50
2 Answers
2
There basically two sides of Unity which are the managed and native side. The managed part is just the C# API and the native side is just the native side of the code written in C++ that communicates with Object-C Swift for iOS or Java for Android to access.
The managed part extension is usually .dll and the native side for Android are usually .so, .aar, .jar or just a .dex.
The managed part extension for iOS native side are usually .a, .m, .mm, .c, .cpp. For iOS, some manage part are not compiled and still in form of .cpp until you build the generated project with xCode.
This code works on Andoid and on iOS. Im wondering, how does Unity
know if we're running the game on Android or iOS?
They don't do this during run-time. There are so many Unity API and doing this every time would be redundant and messy.
To simplify this, it uses different managed and native files for different platforms. These files are included during build-time and this decision is made in the Editor once you click the build button.
First, go to <UnityInstallationDirecory>EditorDataPlaybackEngines
<UnityInstallationDirecory>EditorDataPlaybackEngines
To avoid using some preprocessor directives, Application.platform
or mising different platforms codes, this is done in the Editor during build time. If you select iOS and build the project, it will include UnityEngine.dll
for iOS located in the C:Program <UnityInstallationDirecory>EditorDataPlaybackEnginesiOSSupport
path. It will do the-same for Android but in the <UnityInstallationDirecory>EditorDataPlaybackEnginesAndroidPlayer
path.
Application.platform
UnityEngine.dll
<UnityInstallationDirecory>EditorDataPlaybackEnginesiOSSupport
<UnityInstallationDirecory>EditorDataPlaybackEnginesAndroidPlayer
There are different UnityEngine.dll for each platform. You can see them in the path I mentioned above. This UnityEngine.dll and other managed dlls is where you will see a call to the native side of the code with DllImport
or with AndroidJavaClass
. Note that UnityEngine.dll is not the only file included in that build. Most files in these paths, with the extensions I mentioned above are included. These includes the managed and native files.
DllImport
AndroidJavaClass
Is there any way I can see where and how we get the data from the Android sensor? Im assuming Unity's class "Input.acceleration" gets data out of Android's class "public static final int TYPE_ACCELEROMETER" (developer.android.com/reference/android/hardware/…). Is this class included anywhere in the <UnityInstallationDirecory>EditorDataPlaybackEnginesAndroidPlayer path?
– kac26
Jul 1 at 21:09
Yes it is but it is not open source. You can't see the source unless you decompile it which is against Unity's terms you agreed. If you work for a company, you can pay Unity to get the source code but it's very expensive.The file is there. For accelerometer, I suspect one of the ".so" or ".a files. For the Input.GetKeyXX functions then it's in the "classes.jar" file at
C:Program FilesUnityEditorDataPlaybackEnginesAndroidPlayerVariationsmonoReleaseClasses
if you de-compile the jar file. You will see the implementation there.– Programmer
Jul 2 at 0:06
C:Program FilesUnityEditorDataPlaybackEnginesAndroidPlayerVariationsmonoReleaseClasses
The proper way to determine what platform you are on would be to check with the #define
directives that Unity uses. You can find more information about it in the docs here
#define
Here is an example of how you can check if you are on Android or IOS:
#if UNITY_ANRDOID
// Android code goes here
#elif UNITY_IOS
// IOS Code goes here
#endif
Hope this helps!
If you want a runtime check, then you can use the RuntimePLatform
if (Application.platform == RuntimePlatform.Android)
value = 5;
else if(Application.platform == RuntimePlatform.IPhonePlayer)
value = 10;
Question is not asking "how to determine the platform.". I think you should read it again
– Programmer
Jul 1 at 16:59
Edited to add a runtime check as well, although the question does ask : Im wondering, how does Unity know if we're running the game on Android or iOS?
– BenjaFriend
Jul 1 at 17:03
When you said "The proper way to determine what platform you are on would be to check with the #define directives that Unity uses." it seems more like you were telling OP how to detect platform instead of answering how unity detects which platform it is to use a different API. The question is asking how one API works on multiple platforms. How Unity determines which API to use on each platform.
– Programmer
Jul 1 at 17:55
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
This may be a shot in the dark, but when your running on Windows you are using the UnityEngine.dll... however I don't believe Android or iOS use the dll... I believe they use .so's or .a's why would you find anything in the UnityEngine.dll for distinguishing the 2 different OS's?
– Eddge
Jul 1 at 16:26