ReactJS - Axios doesn't return data
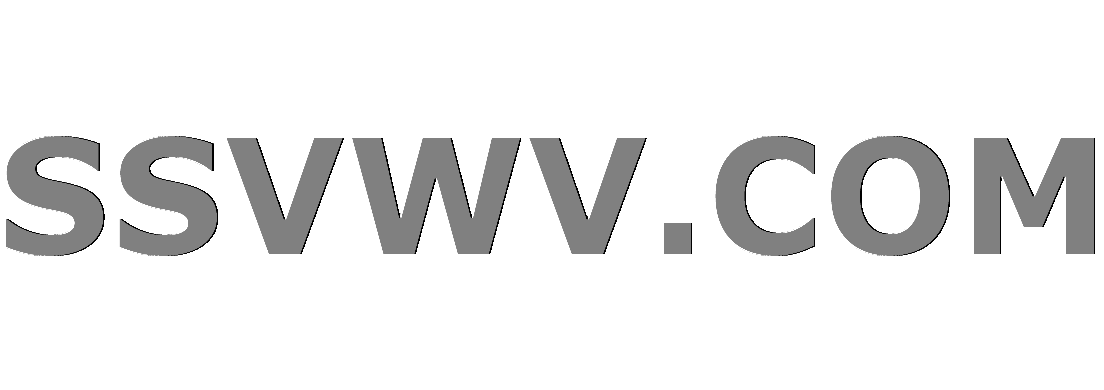
Multi tool use
ReactJS - Axios doesn't return data
I have this code to get data with Axios. Unfortunately, this code is not working because the console.log(response);
is not logging any data in the console. What is wrong here? Is there something missing?
console.log(response);
const ROOT_URL = "https://jsonplaceholder.typicode.com";
const getFindAll = (data) => {
return {
type: constants.FETCH_FIND_ALL,
payload: data
}
}
export const fetchFindAll = () => {
return (dispatch) => {
axios({
method: 'put',
url: `${ROOT_URL}/posts/1`
})
.then(response => {
console.log(response);
dispatch(getFindAll(response.data.Body.Message));
})
.catch(error => {
//TODO: handle the error when implemented
})
}
}
I'm calling the fetchFindAll()
inside FileTree
component:
fetchFindAll()
FileTree
export class FileTree extends React.Component {
constructor(props) {
super(props);
this.state = { activeNode: null };
console.log('ldirj', props)
this.setActiveNode = this.setActiveNode.bind(this);
}
setActiveNode(name) {
this.setState({ activeNode: name });
this.props.liftStateUp(name);
}
componentWillReceiveProps({ searchTerm }) {
this.setState({ searchTerm });
}
renderFindAll () {
const fetch = this.props.fetchFindAll();
fetch(this.props.dispatch);
console.log(fetch);
}
componentDidMount () {
this.renderFindAll();
}
render() {
return (
<div className="grid__item-50">
{renderTree(this.props.root, this.setActiveNode, this.state.activeNode, null, this.state.searchTerm)}
</div>
);
}
}
//TODO: handle the error when implemented
Look at the Console. It might not log the data, but it might log some other error message.
– Quentin
Jul 1 at 16:22
Look at the Network Tab in the browser's developer tools. Is the request sent? Is the request formatted the way you expect? Does it get a response? Is the response the one you expect?
– Quentin
Jul 1 at 16:23
@Quentin I checked the network tab, and there is nothing there related to the request I trying to do.
– RCohen
Jul 1 at 16:24
@Quentin and I found no errors :/
– RCohen
Jul 1 at 16:25
3 Answers
3
Calling fetchFindAll()
will give you a closure function.
fetchFindAll()
To actually trigger the request, you need to invoke it with fetchFindAll()(dispatch)
fetchFindAll()(dispatch)
fetchFindAll
does not accept any argument, therefore, passing this.state
to it won't make any difference.
fetchFindAll
this.state
Also, calling fetchFindAll
multiple times will give you multiple closure functions.
fetchFindAll
In conclusion, you should do something like this:
renderFindAll () {
const fetch = this.props.fetchFindAll();
fetch(this.props.dispatch); // assuming you are using react-redux
}
---- EDIT ----
Try to implement componentDidMount
:
componentDidMount
componentDidMount () {
this.renderFindAll();
}
I have updated the code with your solution, and it's still not working, unfortunately. @kit
– RCohen
Jul 1 at 17:45
But yes, I am using
react-redux
; @kit– RCohen
Jul 1 at 17:48
react-redux
You should not put
renderFindAll
in render
, you are just rendering it to your DOM, not calling it. If you want to trigger it as soon as the component is rendered, you need to implement componentDidMount
in FileTree
.– kit
Jul 1 at 17:50
renderFindAll
render
componentDidMount
FileTree
You are right.
renderFindAll
inside render()
was just for testing. Even so, console.log(response)
inside fetchFindAll()
is not even logging. This means axios is not getting anything, correct? @kit– RCohen
Jul 1 at 17:55
renderFindAll
render()
console.log(response)
fetchFindAll()
The request doesn't appear inside the Network tab, on dev tools. @kit
– RCohen
Jul 1 at 17:56
this.props.fetchFindAll(this.state)
Calling this function does nothing except return a function ((dispatch) => { ... }
) (which you ignore).
(dispatch) => { ... }
You need to either call the returned function or rewrite fetchFindAll
so it does the work of (dispatch) => { ... }
itself.
fetchFindAll
(dispatch) => { ... }
The latter option is probably the better one, as you aren't passing any variables that you could be closing over.
I called the returned function, but it's still not returning any data. @Quentin
– RCohen
Jul 1 at 16:50
According to your latest edit, you now don't even call
fetchFindAll
let alone do anything with its return value.– Quentin
Jul 1 at 16:54
fetchFindAll
Then this means I didn't completely understood your answer. My apologies.
– RCohen
Jul 1 at 16:56
Okay, it sounds like some react/react-redux things are tripping you up here. I had some time and so I went ahead and modeled how this might work together here:
https://codesandbox.io/s/8n9vvz6lx9
(I didn't setup any reducers/state vars - you should see the response in devtools - console)
I'll try not to go in detail on info you can find in the docs (which are a really great resource, along with the tutorials you can find linked there).
Redux docs: https://redux.js.org/
If you have trouble understanding the react component architecture, react docs & tutorials are plenty as well. (https://reactjs.org/) I assume you're already looking at these.
Redux is really powerful but can be a bit confusing, so I'll attempt to detail the things I've noticed in the code you've provided (keeping in mind that you might know some of these things and you may not have included them in your code here)
1: dispatch
needs to come from somewhere, react-redux offers connect
(https://github.com/reduxjs/react-redux):
dispatch
connect
import { connect } from "react-redux";
// ...
const mapDispatchToProps = (dispatch, ownProps) => ({
dispatch
});
export default connect(null, mapDispatchToProps)(FileTree);
2: Your action should also be imported somewhere:
import { fetchFindAll } from "../actions";
// ...
renderFindAll() {
const fetch = fetchFindAll();
fetch(this.props.dispatch);
console.log(fetch);
}
3: For most components, you want to export default
depending on how you plan to import.
export default
4: In general you can use a put method here, but you're probably more likely wanting to use axios.get('${URL}')
axios.get('${URL}')
5: Take a look at the response data you're getting (using a put request):
Inside of response.data
is {id: 1}
so calling response.data.Body.Message
will error. It's very likely that you wanted to use a get request here which would give you this:
response.data
{id: 1}
response.data.Body.Message
which still doesn't have a response.data.Body.Message
, but maybe that's closer to what you intended.
response.data.Body.Message
Okay, really hope this helps! Good luck!
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Replace
//TODO: handle the error when implemented
with something that handles errors– Quentin
Jul 1 at 16:22