Recursively calculate the sum of an Array of integers in JavaScript
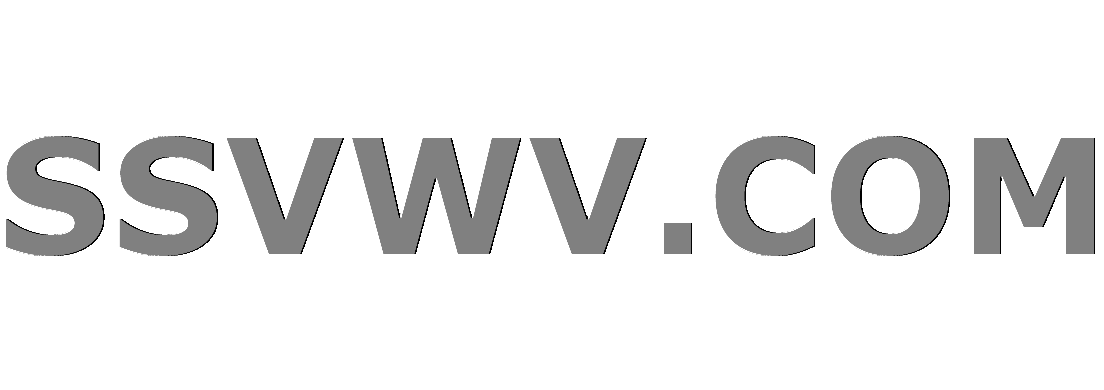
Multi tool use
Recursively calculate the sum of an Array of integers in JavaScript
I wanted to write a JavaScript program to compute the sum of an array of integers Recursively.
Expected Results
Input: [1, 2, 3, 4, 5, 6]
Output: 21
I achieved the above results with this code:
function calculateSum(array) {
if (array instanceof Array){
if (!array.some(isNaN)) {
var total = 0;
array.forEach(function (value) {
total += value;
});
return total;
}
return "Provide an Array with only Numeric Values";
}
return "Please provide an Array";
}
But I'm looking for a solution that uses Recursion.
EDIT: I started doing the above exercise to practice Recursion. I was having a hard time figuring that out. So, That's why I posted this. I'd be glad if you understood.
Thanks in advance.
@Carcigenicate I want a solution that uses Recursion
– Thawindu Angesh Tuto
Jul 1 at 16:10
Yes. Where are you stuck though? Asking to supply an entire solution is too broad. Show your attempt and ask a specific question regarding where you're stuck.
– Carcigenicate
Jul 1 at 16:12
Why recursion when
.reduce()
can easily do what you want?– Terry
Jul 1 at 16:36
.reduce()
4 Answers
4
To use recursion you simply need a base case and way to spilt the input into something smaller you can recurse with.
The sum of an array of length 1 is just arr[0]
right? So that's a plausible base case. With a larger array, the sum is one element plus the sum of all the others. So that's your other case: arr[0] + sum(everything else)
arr[0]
arr[0] + sum(everything else)
Now you can write a simple function with just those two cases:
let arr = [1, 2, 3, 4, 5, 6]
function add(arr) {
if (arr.length == 1) return arr[0] // base case
return arr[0] + add(arr.slice(1)) // recurse
}
console.log(add(arr))
The idea is simple enough that you can express it as a one-liner:
const add = (arr) => arr.length == 1 ? arr[0] : arr[0] + add(arr.slice(1))
console.log(add([1, 2, 3, 4, 5, 6] ))
Of course, you might want better error checking, but this should get you started.
Thanks! This is exactly what I was looking for. 🙏
– Thawindu Angesh Tuto
Jul 1 at 16:49
The recursion is actually a regression problem,
If the array named 'Arr' has only one element - this is the sum,
Now imagine you know the sum formula for an array of N elements,
You can now use recursion to find the sum of the (N+1) elements array since
it is simply the last element plus the sum of the previous N, which you already know/calculated.
An example is attached.
Read more at wikipedia.
let arr = [10,100,1000,10000];
function sum(array){
if(array.length === 1){
return array[0];
}else{
return array[array.length-1] + sum(array.slice(0,array.length-1));
}
}
console.log(sum(arr));
Destructuing syntax permits an elegant functional expression
const None =
Symbol ()
const sum = ([ n = None, ...rest ]) =>
n === None
? 0
: n + sum (rest)
console.log
( sum () // 0
, sum ([ 1 ]) // 1
, sum ([ 1, 2 ]) // 3
, sum ([ 1, 2, 3 ]) // 6
, sum ([ 1, 2, 3, 4 ]) // 10
)
var arr = [1, 2, 3, 4, 5];
function add(arr) {
if(arr.length>1) {
arr[0] += arr.splice(1,1)[0];
return add(arr);
} else
return arr[0];
}
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
What have you tried? What specifically do you need help with?
– Carcigenicate
Jul 1 at 16:10