Am I going down the right path? - Programming Beginner [closed]
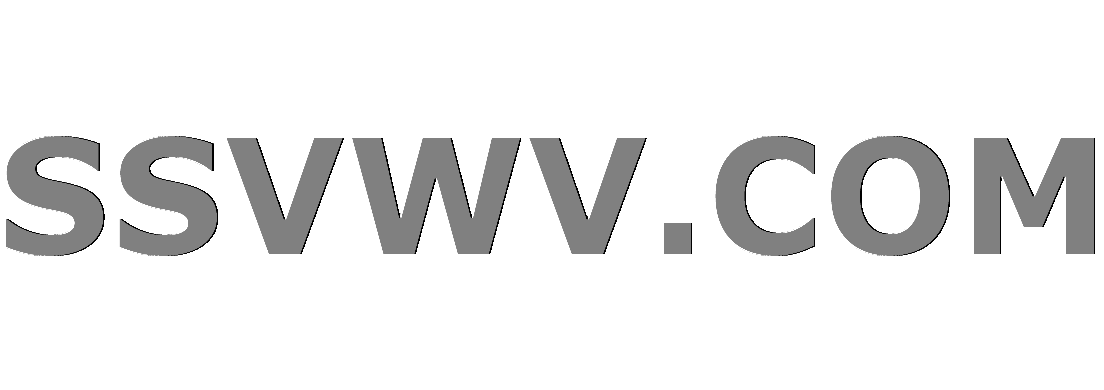
Multi tool use
Am I going down the right path? - Programming Beginner [closed]
Okay so, I am basically a beginner to Java and only recently started to code in Java for a school project of which I have to complete by the end of 2019 or so. Although I have dug right into the development of the game, as I started to code the menu for my game all I knew about Java was how encapsulation, inheritance and constructors work. As a result it was very hard for me to code my menu, I went through multiple youtube videos only to find one that explained it simply for beginners like me eventually leading me to finishing the menu for my game. If I was to code the menu for my game again I feel as if I could not code it.
Is this how I should start off coding? (If you have any useful starting websites please let me know, and the course name if you know a helpful one in particular)
Is it expected for beginners to code a game from scratch?
Is it ideal for me to code the entirety of my game using tutorials?
What would be the best way to learn different features that can be used throughout Java? such as JFrame, Graphics etc.
Here is the entirety of the code that makes my Menu: (If you know of anyway I can improve this, please let me know). Thank you!
Menu Class:
import java.awt.Color;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.Rectangle;
public class menu {
public static Rectangle startButton = new Rectangle(380, 200, 100, 37);
public static Rectangle quitButton = new Rectangle(380, 304, 100, 37);
public static void render(Graphics g) {
Graphics2D graphics2d = (Graphics2D) g;
Font font0 = new Font("arial", Font.BOLD, 105);
Font font1 = new Font("arial", Font.BOLD, 30);
g.setFont(font0);
g.setColor(Color.black);
g.drawString("Game Title", 220, 100);
g.setFont(font1);
g.setColor(Color.white);
g.drawString("Play", startButton.x + 19, startButton.y + 29);
graphics2d.draw(startButton);
g.drawString("Quit", quitButton.x + 19, quitButton.y + 29);
graphics2d.draw(quitButton);
}
}
Frame Class:
import javax.swing.JFrame;
public class frame {
public static void main(String args) {
JFrame frame = new JFrame();
frame.setVisible(true);
frame.setResizable(false);
frame.getContentPane().add(new board());
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(900,613);
frame.setLocation(50, 50);
}
}
Board Class:
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class board extends JPanel {
Image background, menuBackground;
private menu Menu;
private frame Frame;
public static enum STATE {M,G};
public static STATE State = STATE.M;
public board() {
ImageIcon i = new ImageIcon("D:\Pictures\CastleBackground.jpg");
menuBackground = i.getImage();
i = new ImageIcon("D:\Pictures\castlebackground.png");
background = i.getImage();
}
public void paintComponent(Graphics g) {
if(State==STATE.G) {
paintComponent(g);
Graphics2D graphics2d = (Graphics2D) g;
} else {
g.drawImage(menuBackground, 0, 0, null);
menu.render(g);
}
}
}
Many good questions generate some degree of opinion based on expert experience, but answers to this question will tend to be almost entirely based on opinions, rather than facts, references, or specific expertise. If this question can be reworded to fit the rules in the help center, please edit the question.
Also check out the Code Review StackExchange site.
– that other guy
Jul 1 at 17:40
I suggest that you 1) go through appropriate tutorials rather than ask overly-broad questions on this site. 2) consider using the JavaFX GUI library, not Swing.
– Hovercraft Full Of Eels
Jul 1 at 17:40
You're making recursive calls to paintComponent within your board class's paintComponent override, a dangerous and likely wrong thing to do.
– Hovercraft Full Of Eels
Jul 1 at 17:39