how to rename object data Response in React
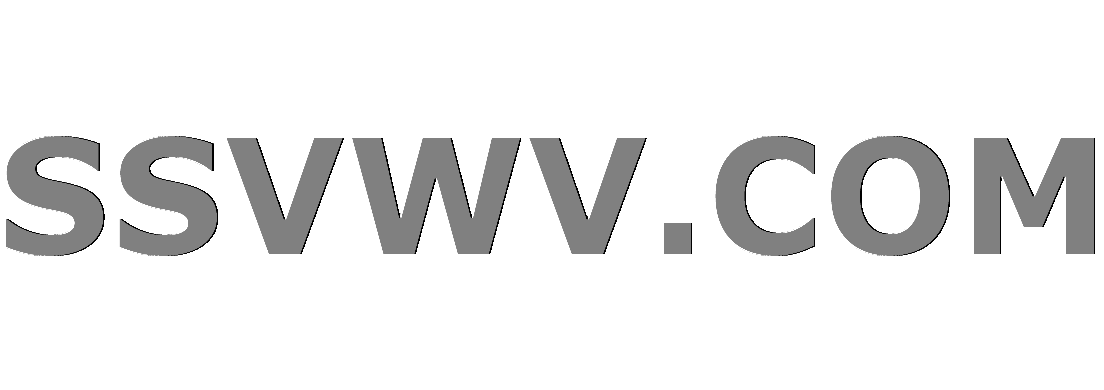
Multi tool use
how to rename object data Response in React
So I'm trying to rename an object property for an array of objects. Here is generally what the response looks like
response: [{
name: 'Manage User',
id: 1,
}, {
name: 'Manage Region',
id: 2,
}, {
name: 'Manage BTP',
id: 3,
}
],
the function
getResponseRename() {
return this.response.map((data) =>
<div>
<p>title: {data.key}</p>
<span>key: {data.title}</span>
<hr/>
</div>
);
}
render(){
return(
<div>{this.getResponseRename()}</div>
)
}
and I would like to change the payload "name" to "title" and "id" to "key". How could I change this and mapping the new response data after rename it?any help would be really appreciate
name
id
title
key
yeah I want to change the actual data
– Satria stuart
Jul 1 at 18:16
2 Answers
2
If I understand your question correctly, you can do this with ES6 destructuring aliases:
getResponseRename() {
return this.response.map(({id: key, name: title}) =>
<div>
<p>title: {key}</p>
<span>key: {title}</span>
<hr/>
</div>
);
}
This will destructure your callback parameter into the id
and name
variables, and assign them a new variable name (aka an alias).
id
name
From the MDN documentation:
A property can be unpacked from an object and assigned to a variable with a different name than the object property.
var o = {p: 42, q: true};
var {p: foo, q: bar} = o;
console.log(foo); // 42
console.log(bar); // true
get your data:
response: [{
name: 'Manage User',
id: 1,
}, {
name: 'Manage Region',
id: 2,
}, {
name: 'Manage BTP',
id: 3,
}],
reformat it:
const reformattedData = response.map((data) => {
return {
title: data.name,
key: data.id,
};
})
use it!
getResponseRename() {
return reformattedData.map((data) =>
<div>
<p>title: {data.key}</p>
<span>key: {data.title}</span>
<hr/>
</div>
);
}
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Do you want to just display
name
andid
astitle
andkey
in the render method, or do you want to change your actual data to those values?– Tholle
Jul 1 at 17:30