Comparable arrays in Kotlin
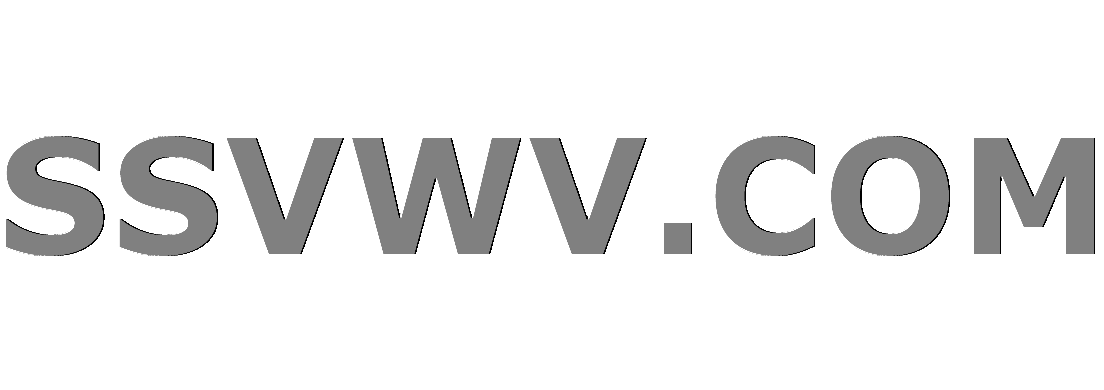
Multi tool use
Comparable arrays in Kotlin
Coming from a Swift world I'm trying to figure out how to use comparable functions like min()
or max()
on an array of objects. In Swift I would use the comparable protocol -
min()
max()
class Car: Comparable {
let year: Int
static func < (lhs: Car, rhs: Car) -> Bool {
return lhs.year < rhs.year
}
static func == (lhs: Car, rhs: Car) -> Bool {
return lhs.year == rhs.year
}
}
But how would you do the same in Kotlin? I've tried this but I'm not sure if it's the right approach, or how I would implement the iterable function -
data class Car(val year: Int): Comparable<Car>, Iterable<Car> {
override fun compareTo(other: Car) = when {
this.year < other.year -> -1
this.year > other.year -> 1
else -> 0
}
override fun iterator(): Iterator<Car> {
TODO("not implemented")
}
}
min
max
Comparable
this.year - other.year
The
compareTo
method looks fine, but you don't need the car to be an Iterable
. That doesn't make any sense.– marstran
Jul 1 at 16:57
compareTo
Iterable
1 Answer
1
Regarding your implementation there's no issue, but you could make it simpler:
data class Car(val year: Int): Comparable<Car> {
override fun compareTo(other: Car) = year - other.year
}
You should not implement the Iterable
interface, because this would make the Car
a kind of a collection.
Iterable
Car
Running the following example with the given Car
implementation
Car
listOf(Car(2004), Car(2007), Car(2001)).run {
println(this)
println(min())
println(max())
println(sorted())
}
yields the output of
[Car(year=2004), Car(year=2007), Car(year=2001)]
Car(year=2001)
Car(year=2007)
[Car(year=2001), Car(year=2004), Car(year=2007)]
Right ok. I think it's the
run
bit I was missing. I was trying to call max()
on the list directly listOf(Car(2004), Car(2007), Car(2001)).max()
which I could only do if I implemented the Iterable
interface.– Chris Edgington
Jul 1 at 17:49
run
max()
listOf(Car(2004), Car(2007), Car(2001)).max()
Iterable
There is actually no difference and your code there runs fine as well. I'd assume you missed a closing brace somewhere and thus the compiler tried to call
max()
on a Car
.– tynn
Jul 1 at 18:03
max()
Car
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
So your end goal is to be able to call
min
andmax
on an array of Cars? You can already do that with yourComparable
implementation, although it could be simpler (this.year - other.year
).– zsmb13
Jul 1 at 16:56