copy single row from multiple worksheets into new worksheet
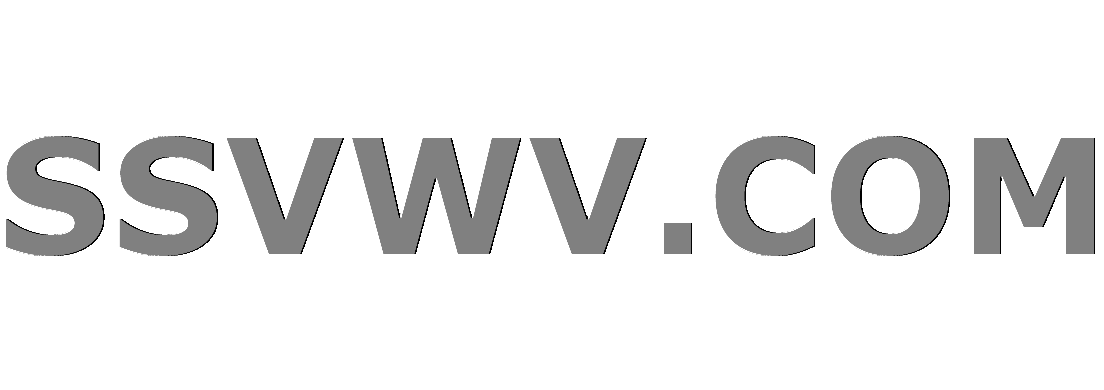
Multi tool use
copy single row from multiple worksheets into new worksheet
I'm not a developer, but was recently hired for a newly created position, meaning I'm trying to create reports and things from scratch that have never been done before. The IT department doesn't have time to teach me and so I'm trying to learn VBA and Access and other advanced data analysis tools, but I don't understand how to write code to the extent I need it yet. I used several things from these forums, but I've been lucky enough to mostly copy and paste to get what I need.
I copied this from this forum (second answer): copy the same row from multiple sheets into one sheet in excel
This is the code I copied:
Sub copyrow()
Dim Nrow As Long, Nsheet As Long
Dim i As Long
Nrow = 7
Nsheet = 6
For i = 1 To Nsheet - 1
Sheets(i).Cells(Nrow, 1).EntireRow.Copy Sheets(Nsheet).Cells(i, 1)
Next i
End Sub
I tested it and it worked, but I didn't realize that Nsheet meant it would go to the 6th sheet and replace the data there. So I began again and opened a blank worksheet at the front of the others. All I did was change Nrow to 6 because that's the row I want copied. Then I changed Nsheet to 1 so it would put the results on the first sheet. Once I made that Nsheet change, it wouldn't work. I don't understand the code enough to know what I did wrong.
Here's what my code looks like now:
Sub copyrow()
Dim Nrow As Long, Nsheet As Long
Dim i As Long
Nrow = 6
Nsheet = 1
For i = 1 To Nsheet - 1
Sheets(i).Cells(Nrow, 1).EntireRow.Copy Sheets(Nsheet).Cells(i, 1)
Next i
End Sub
But when I run it, nothing happens. I currently have the code pasted into the first worksheet. I thought maybe I should add the code to another worksheet but that gave me an error when trying to run. I don't know if that matters. I feel really stupid and am sure it's a minor petty thing, so feel free to roll your eyes.
Thanks!
The code should go into a public module, not a worksheet's private code sheet. Open the VBE with alt+F11 then Insert, Module and paste your code there. Remove it from everywhere else.
– Jeeped
Jul 1 at 17:04
David, okay, yes, that makes sense. I was just learning about For loops. For this purpose, the data will always be on the first sheet and will always be gathered from the 6 following sheets in the workbook. My hope is to create this "Overview sheet," save it as a template, and then rerun it (I have a little more than 50 separate workbooks that I need to do this for.
– Kat
Jul 1 at 17:19
@Jeeped, thank you, I did that. I didn't know that it needed to be in a Module. There is still something wrong with the code, but I'm determined to figure it out. Thanks!
– Kat
Jul 1 at 17:34
2 Answers
2
Loop from the 2nd to the 6th sheet to gather data. Nsheet is now changed back to 6 and your loop increases i from 2 to 6. It works the same if you could remove the 'Nsheet' assignment and change your For loop to For i = 2 to 6
.
For i = 2 to 6
If the number of sheets changes for each spreadsheet, you could use Sheets.Count
instead of specifying 6
.
Sheets.Count
6
In .Cells(i - 1, 1)
... the -1
has been added because i
is reused. i
is used as a sheet reference when looping through sheets to gather data; here, it is reused as a row number variable. Sheet2 data will be added to row 1 of sheet1, sheet3 data will be added to row 2 of sheet1, etc. If there are headers on your sheet1, then -1
can be removed.
.Cells(i - 1, 1)
-1
i
i
-1
Sub copyrow()
Dim Nrow As Long, Nsheet As Long
Dim i As Long
Nrow = 7
Nsheet = 6
For i = 2 To Nsheet
Sheets(i).Cells(Nrow, 1).EntireRow.Copy Sheets(1).Cells(i - 1, 1)
Next i
End Sub
I know I'm not supposed to comment with "thanks," but thank you! I actually changed the value to +2 because I have two rows of headers. What if I wanted to paste the row from each sheet into a specific row in Sheet1? For example, row6 from Sheet2 into the 3rd row, row6 from Sheet2 into the 5th row, etc. is that possible? This is perfect, but I'm just curious for future reference.
– Kat
Jul 1 at 17:46
Great, you're welcome and thanks for the feedback :-). To paste into specific rows on your result sheet... if there is a pattern to those rows (I mean if you literally examine the numbers and there's a pattern; a relationship) then you can try to build the logic into your loop. But if there's no obvious pattern then, since you're only gathering data from 5 sheets, you could get rid of the loop and replace the
i
variable with specific numbers on the Sheets(i).Cells...
line, and repeat that line for every sheet you're gathering from.– David
Jul 1 at 17:59
i
Sheets(i).Cells...
Dim Nrow As Long, Nsheet As Long
Dim i As Long
You're on the right track here. Always use Long; never use Integer. Integers aren't cool and will invisibly break your code faster than anything else. Longs are cool and won't break your code for silly reasons.
... and opened a blank worksheet at the front of the others.
Your code can do this easily.
worksheets.add(before:=worksheets(1)).name = "All Rows"
If you run this a second time, you will have to change the name or first remove the existing worksheet named 'All Rows'.
Nrow = 7
Nsheet = 6
You want to copy row 6 from all other worksheets so NRow = 6. Nsheet can be used to hold the total number of worksheets in the workbook.
Nrow = 6
Nsheet = worksheets.count
For i = 1 To Nsheet - 1
...
Next i
You created a new worksheet at the beginning of the queue so to address the other worksheets, start at 2. Loop through until you reach the total number of worksheets, i.e. Nsheet as was assigned above.
for i=2 to Nsheet
' <copying code goes here>
next i
Sheets(i).Cells(Nrow, 1).EntireRow.Copy Sheets(1).Cells(i - 1, 1)
This should work 'as-is' now. I prefer to use the Worksheets collection instead of the Sheets collection since a Chart page, Macro sheet, etc is a member of Sheets but not of Worksheets. I also typically add the Destination parameter name since it makes the code easier to read. You might want to use column letters instead of the column ordinal index number for clarity.
Worksheets(i).Cells(Nrow, "A").EntireRow.Copy Destination:=Worksheets(1).Cells(i - 1, "A")
Put this altogether in a public module code sheet, not a worksheet's private code sheet. Private code sheets have their purpose, typically for code that primarily involves that worksheet only. This code is just better suited to a public module.
Sub copyrow()
Dim Nrow As Long, Nsheet As Long
Dim i As Long
worksheets.add(before:=worksheets(1)).name = "All Rows"
Nrow = 6 'row to copy
Nsheet = worksheets.count 'the count AFTER adding the destination worksheet
For i = 2 To Nsheet
Worksheets(i).Cells(Nrow, "A").EntireRow.Copy Destination:=Worksheets(1).Cells(i - 1, "A")
Next i
End Sub
Indent your code for readability.
Go into the VBE's Tools, Options and put a check beside Require variable declaration. This will put Option Explicit
at the top of every new code sheet you create/insert. It will save you hours of grief from silly variable misspellings or omissions. It is also quite possibly the single biggest time saver that you will add to your coding experience.
Option Explicit
This was very helpful and informative. The insert worksheet code was genius because it doesn't overwrite any of my data so when I mess up my code (I'm still trying to tweak further to make it do exactly what I want- you guys made me feel successful, so I'm looking for further ways to automate some of the processes), I can just delete the whole sheet. As for using worksheets instead of sheets, if I eventually want to pull in data from a pivot table, does that mean I'll use sheets instead? Also, I have no idea what variable declaration does but I went in and checked it anyway. Thanks!
– Kat
Jul 1 at 20:54
A pivot table 'sheet' is still a worksheet. Glad to help out. btw, you're a Developer now.
– Jeeped
Jul 1 at 21:01
Declarations means if you use i then you have to declare i (e.g. Dim i as long). If you declared Nsheet with dim NSheet as long but later tried to use nshet, Option Explicit will tell you that you have an undeclared var. fwiw, we get questions regarding code that won't work due to a misspellling several times a week around here.
– Jeeped
Jul 1 at 21:05
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
I'll explain a little about the 'For' loop and then you'll need to give us a little extra information about your spreadsheet. The 'For' loop uses the variable i and increases it by 1 with each loop. For instance, a For loop could start "For i = 1 to 10" and on the first iteration i would be equal to 1; on the second iteration i would be equal to 2 and so on. Your For loop is designed to loop through sheets in your workbook. Instead of specifying a number, it can be dynamic. Will your results sheet always be the first sheet? Do you want to gather data from all other sheets?
– David
Jul 1 at 17:03