Android 6 : Connect to specific wifi network programmatically not working
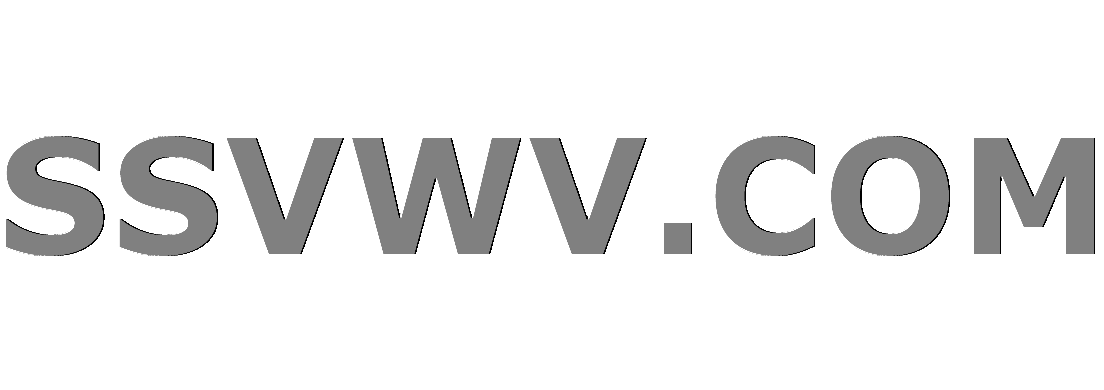
Multi tool use
Android 6 : Connect to specific wifi network programmatically not working
I am trying to connect to a wifi network by giving the SSID and pass using WifiManager configurations.
Based on this threads solution:
How do I connect to a specific Wi-Fi network in Android programmatically?
The reconnect method is called. but nothing happens (not connected).
Is the Android version (6.0.1) for something?
If yes then how to perform a network connection programmatically on Android 6?
6 Answers
6
A few things have changed about how you connect to a WiFi network since android Marshmallow.
The following code will help you...If you are using Android 6.0 or lowlevel versions...
public void connectToWifi(){
try{
WifiManager wifiManager = (WifiManager) super.getSystemService(android.content.Context.WIFI_SERVICE);
WifiConfiguration wc = new WifiConfiguration();
WifiInfo wifiInfo = wifiManager.getConnectionInfo();
wc.SSID = ""NETWORK_NAME"";
wc.preSharedKey = ""PASSWORD"";
wc.status = WifiConfiguration.Status.ENABLED;
wc.allowedProtocols.set(WifiConfiguration.Protocol.WPA);
wc.allowedKeyManagement.set(WifiConfiguration.KeyMgmt.WPA_PSK);
wifiManager.setWifiEnabled(true);
int netId = wifiManager.addNetwork(wc);
if (netId == -1) {
netId = getExistingNetworkId(wc.SSID);
}
wifiManager.disconnect();
wifiManager.enableNetwork(netId, true);
wifiManager.reconnect();
} catch (Exception e) {
e.printStackTrace();
}
}
private int getExistingNetworkId(String SSID) {
WifiManager wifiManager = (WifiManager) super.getSystemService(Context.WIFI_SERVICE);
List<WifiConfiguration> configuredNetworks = wifiManager.getConfiguredNetworks();
if (configuredNetworks != null) {
for (WifiConfiguration existingConfig : configuredNetworks) {
if (existingConfig.SSID.equals(SSID)) {
return existingConfig.networkId;
}
}
}
return -1;
}
And add permissions in the Manifest file
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />
<uses-permission android:name="android.permission.CHANGE_WIFI_STATE" />
<uses-permission android:name="android.permission.CHANGE_NETWORK_STATE" />
not working to me
– kemdo
Mar 26 at 6:41
Add a wifi configuration using addNetwork and then connect to it using enableNetwork.
WifiConfiguration wificonfiguration = new WifiConfiguration();
StringBuffer stringbuffer = new StringBuffer(""");
stringbuffer.append((new StringBuilder(String.valueOf(HOTSPOT_NAME))).append(""").toString());
wificonfiguration.SSID = stringbuffer.toString();
wificonfiguration.allowedKeyManagement.set(KeyMgmt.WPA_PSK);
wificonfiguration.allowedAuthAlgorithms.set(0);
wificonfiguration.status = 2;
wificonfiguration.preSharedKey = """ + HOTSPOT_PASSWORD + """;
int networkId_ = wifi.addNetwork(wificonfiguration);
if(networkId>-1){
boolean status = wifi.enableNetwork(networkId_, true);
}
For marshmallow: Your apps can now change the state of WifiConfiguration objects only if you created these objects. You are not permitted to modify or delete WifiConfiguration objects created by the user or by other apps.
More info on Marshmallow
HI, that line solved my problem : wificonfiguration.allowedKeyManagement.set(KeyMgmt.WPA_PSK); Now the connection is working. Thanks
– chimaira
Mar 13 '16 at 14:06
this doenst work in android 6+
– xanexpt
Jul 20 '16 at 13:23
@xanexpt: Did you get it working for android 6 by any chance?
– user2489122
Jan 23 '17 at 6:58
A few things have changed about how you connect to a WiFi network since android Lollipop and Marshmallow. There is a nice blog post related to how you connect to WiFi network on Marshmallow and below versions
http://www.intentfilter.com/2016/08/programatically-connecting-to-wifi.html
The post describes a scenario when WiFi network has no connectivity and you want to send traffic through that network (something like captive portal). But it also explains the complete process and would work for normal networks. You won't find the exact code but it might still help in knowing where you got stuck.
You need to call disconnect() and reconnect() of WiFiManager. This works for me:
WifiConfiguration conf = new WifiConfiguration();
conf.hiddenSSID = true;
conf.priority = 1000;
conf.SSID = """ + SSID + """;
conf.preSharedKey = """+Password+""";
conf.status = WifiConfiguration.Status.ENABLED;
conf.allowedAuthAlgorithms.set(WifiConfiguration.AuthAlgorithm.OPEN);
conf.allowedGroupCiphers.set(WifiConfiguration.GroupCipher.WEP40);
conf.allowedGroupCiphers.set(WifiConfiguration.GroupCipher.WEP104);
conf.allowedGroupCiphers.set(WifiConfiguration.GroupCipher.TKIP);
conf.allowedGroupCiphers.set(WifiConfiguration.GroupCipher.CCMP);
conf.allowedKeyManagement.set(WifiConfiguration.KeyMgmt.WPA_PSK);
conf.allowedKeyManagement.set(WifiConfiguration.KeyMgmt.WPA_EAP);
conf.allowedPairwiseCiphers.set(WifiConfiguration.PairwiseCipher.TKIP);
conf.allowedPairwiseCiphers.set(WifiConfiguration.PairwiseCipher.CCMP);
conf.allowedProtocols.set(WifiConfiguration.Protocol.WPA);
conf.allowedProtocols.set(WifiConfiguration.Protocol.RSN);
int res = wifiManager.addNetwork(conf);
boolean es = wifiManager.saveConfiguration();
Log.d(TAG, "saveConfiguration returned " + es );
wifiManager.disconnect();
boolean bRet = wifiManager.enableNetwork(res, true);
Log.i(TAG, "enableNetwork bRet = " + bRet);
wifiManager.reconnect();
but how we will find that its connecting, or got any password error?
– NehaK
Dec 1 '17 at 18:15
It still always connects to any previous closest network, not matter which new SSID you add / select. EDIT: Using @Nishkarsh's priority method resolves this problem
– behelit
Jan 16 at 23:28
This works for me for a WPA connection.
You don't need to add again a network if it is already saved.
private void connectToWifi(final String networkSSID, final String networkPassword) {
int netID = -1;
String confSSID = String.format(""%s"", networkSSID);
String confPassword = String.format(""%s"", networkPassword);
netID = getExistingNetworkID(confSSID, netID);
/*
* If ssid not found in preconfigured list it will return -1
* then add new wifi
*/
if (netID == -1) {
Log.d(TAG,"New wifi config added");
WifiConfiguration conf = new WifiConfiguration();
conf.SSID = confSSID;
conf.preSharedKey = confPassword;
netID = wifiManager.addNetwork(conf);
}
wifiManager.disconnect();
wifiManager.enableNetwork(netID, true);
wifiManager.reconnect();
}
private int getExistingNetworkID (String confSSID, int netID){
List<WifiConfiguration> wifiConfigurationList = wifiManager.getConfiguredNetworks();
for (WifiConfiguration item : wifiConfigurationList){
/*
Find if the SSID is in the preconfigured list - if found get netID
*/
if (item.SSID != null && item.SSID.equals(confSSID)){
Log.d(TAG, "Pre-configured running");
netID = item.networkId;
break;
}
}
return netID;
}
Try this and enjoy:
int res = mWifiManager.addNetwork(wifiConfiguration);
if (res == -1) {
// Get existed network id if it is already added to WiFi network
res = getExistingNetworkId(wifiConfiguration.SSID);
Log.d(TAG, "getExistingNetwrkId: " + res);
}
private int getExistingNetworkId(String SSID) {
List<WifiConfiguration> configuredNetworks = mWifiManager.getConfiguredNetworks();
if (configuredNetworks != null) {
for (WifiConfiguration existingConfig : configuredNetworks) {
if (SSID.equalsIgnoreCase(existingConfig.SSID)) {
return existingConfig.networkId;
}
}
}
return -1;
}
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Did you get solution?
– SKK
Jul 19 '16 at 14:32