Different look/style for specific item on BottomNavigationMenu
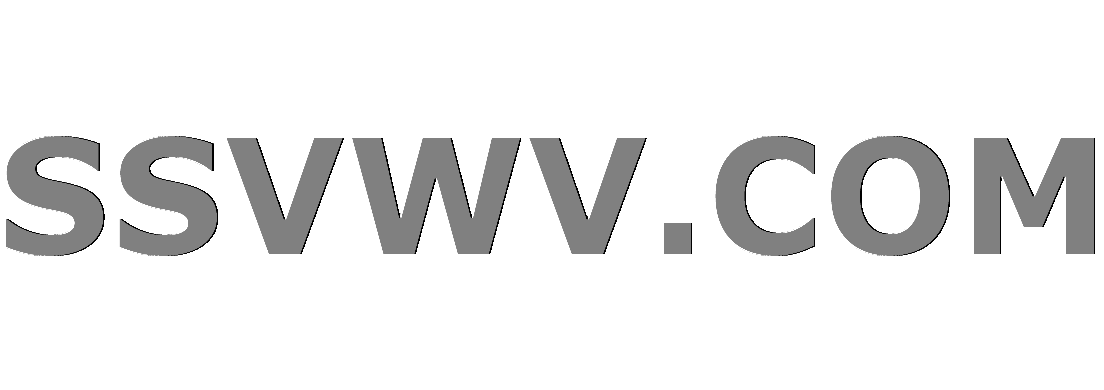
Multi tool use
Different look/style for specific item on BottomNavigationMenu
I am currently building a Xamarin Android application, and I am trying to customize the BottomNavigationView.
I would like to be able to change the style of a specific menu item in the BottomNavigationView from the following:
To this style:
I have had a look at the following question: Different look/style for specific menu item on ActionBar but it seems more targeted towards the action bar rather then the bottom navigation.
I'm guessing this would either need a custom control implemented or use of reflection to achieve the desired result? Any third-party libraries that implement this sort of functionality, aren't particularly helpful as majority of them are written for native android and not xamarin.
Any help in either Xamarin C# or Native Java (that I could convert) would be great thanks.
Currently I tried setting a custom action layout on the menu item programmatically:
var nav = FindViewById<BottomNavigationView>(R.Id.bottom_navigation);
var result = nav.Menu.GetItem(2);
result.SetTitle(null);
result.SetIcon(null);
var image = new ImageButton(this);
image.SetImageDrawable(GetDrawable(R.Drawable.ic_action_grade));
image.SetColorFilter(Android.Graphics.Color.Black);
result.SetActionView(image);
And by specifying a custom layout, using the menu item's app:actionLayout
property. Source: https://www.techrepublic.com/article/pro-tip-use-a-custom-layout-to-badge-androids-action-bar-menu-items/
app:actionLayout
view_accent_grade.axml (Action Layout)
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@android:color/black">
<ImageView
android:id="@+id/ic_action_grade"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_gravity="center"
android:src="@drawable/ic_action_grade" />
</RelativeLayout>
menu.axml (Menu Layout)
<?xml version="1.0" encoding="utf-8"?>
<menu
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="https://schemas.android.com/apk/res-auto">
<item
android:id="@+id/navigation_home"
android:title="@string/action_home"
android:icon="@drawable/ic_action_home" />
<item
android:id="@+id/navigation_events"
android:title="@string/action_events"
android:icon="@drawable/ic_action_event" />
<item
android:id="@+id/navigation_car_of_the_month"
app:showAsAction="ifRoom"
app:actionLayout="@layout/view_accent_grade" /> <!-- Specifying App Layout here -->
<item
android:id="@+id/navigation_photos"
android:title="@string/action_photos"
android:icon="@drawable/ic_action_photo" />
<item
android:id="@+id/navigation_more"
android:title="@string/action_more"
android:icon="@drawable/ic_action_more_horiz" />
</menu>
@G.hakim please have a look at my updated answer
– Harry James Sanderson
Jun 29 at 12:55
the code you are using is not giving you the desired result but what exactly is it doing so far ?
– G.hakim
Jun 29 at 13:07
@G.hakim currently both approaches are creating the menu item, but neither are displaying the actionLayout.
– Harry James Sanderson
Jun 29 at 13:15
you want the star to look like in the second image?
– G.hakim
Jun 29 at 13:21
1 Answer
1
You are probably better off writing a custom bottom navigation view yourself instead of trying to work in the constraints of the BottomNavigationView
control. Here is a quick example:
BottomNavigationView
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/container"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/grey_5">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:orientation="vertical">
<View
android:layout_width="match_parent"
android:layout_height="4dp"
android:background="@drawable/bg_gradient_soft" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="50dp"
android:background="@android:color/white"
android:orientation="horizontal">
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:gravity="center"
android:padding="@dimen/spacing_medium">
<ImageButton
android:layout_width="?attr/actionBarSize"
android:layout_height="?attr/actionBarSize"
android:background="?attr/selectableItemBackgroundBorderless"
android:onClick="clickAction"
android:tint="@color/teal_600"
app:srcCompat="@drawable/ic_apps" />
</LinearLayout>
<View
android:layout_width="?attr/actionBarSize"
android:layout_height="0dp" />
<LinearLayout
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"
android:gravity="center"
android:padding="@dimen/spacing_medium">
<ImageButton
android:layout_width="?attr/actionBarSize"
android:layout_height="?attr/actionBarSize"
android:background="?attr/selectableItemBackgroundBorderless"
android:onClick="clickAction"
android:tint="@color/teal_500"
app:srcCompat="@drawable/ic_local_offer" />
</LinearLayout>
</LinearLayout>
</LinearLayout>
<android.support.design.widget.FloatingActionButton
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_centerInParent="true"
android:layout_marginBottom="15dp"
android:clickable="true"
android:onClick="clickAction"
android:tint="@android:color/white"
app:backgroundTint="@color/teal_500"
app:elevation="2dp"
app:fabSize="normal"
app:rippleColor="@color/deep_orange_400"
app:srcCompat="@drawable/ic_shopping_cart" />
</RelativeLayout>
Result:
Otherwise you can traverse the layout and apply whatever view styling you want to BottomNavigationView
like the following answer:
BottomNavigationView
Changing BottomNavigationView's Icon Size
EDIT:
There's a binding of an extension library to BottomNavigationView
that has a sample of what you're trying to do:
BottomNavigationView
https://github.com/NAXAM/bottomnavigationviewex-android-binding
Thanks I will give this a try tomorrow! The bottom navigation view does seem to be incredibly limited.
– Harry James Sanderson
Jul 2 at 16:50
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
show me what have you done so far
– G.hakim
Jun 29 at 10:40