How to use C++ /WinRT HttpRequestMessage with Custom POST Body and HttpFormUrlEncodedContent (UWP App)
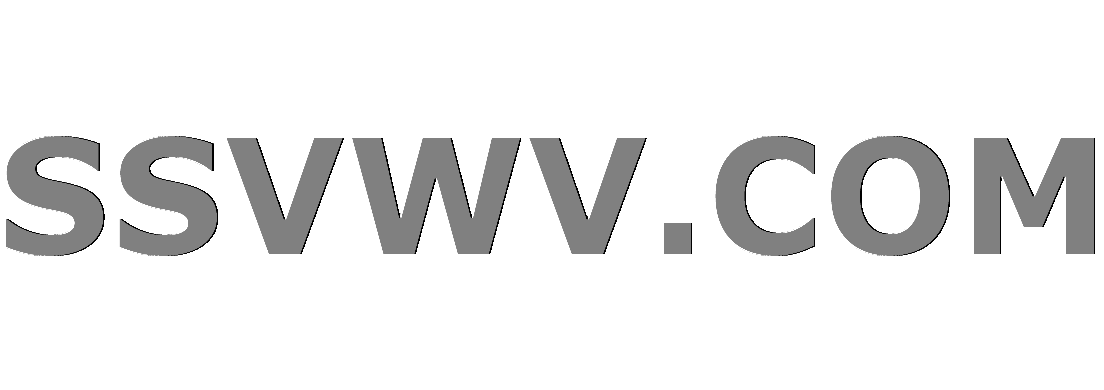
Multi tool use
How to use C++ /WinRT HttpRequestMessage with Custom POST Body and HttpFormUrlEncodedContent (UWP App)
In Windows 10, 1803 it is possible to call the WinRT from standard conforming C++. The C++ /CX language extensions are not required anymore.
I already know how to send a post Request to a Server using the HttpRequestMessage Class:
#pragma comment(lib, "windowsapp")
#include "pch.h"
#include "winrt/Windows.Web.Http.Filters.h"
#include "winrt/Windows.Web.Http.Headers.h"
#include <winrt/Windows.Foundation.h>
#include <winrt/Windows.Foundation.Collections.h>
#include <string_view>
using namespace winrt;
using namespace Windows::Foundation;
using namespace Windows::Web::Http;
using namespace Windows::Web::Http::Filters;
using namespace Windows::Web::Http::Headers;
using namespace Windows::Foundation::Collections;
using namespace std::literals;
using namespace std;
IAsyncAction HttpClientExample(Uri uri)
{
HttpBaseProtocolFilter filter;
HttpClient httpClient(filter);
HttpRequestMessage loginRequest(HttpMethod::Post(), uri);
try
{
auto response{ co_await httpClient.SendRequestAsync(loginRequest) };
HttpCookieCollection cookieCollection = filter.CookieManager().GetCookies(uri);
wcout << cookieCollection.Size() << L" cookies found." << endl;
for (HttpCookie cookie : cookieCollection) {
wcout << L"Name: " << cookie.Name().c_str() << endl;
wcout << L"Domain: " << cookie.Domain().c_str() << endl;
wcout << L"Path: " << cookie.Path().c_str() << endl;
wcout << L"Value: " << cookie.Value().c_str() << endl;
}
}
catch (winrt::hresult_error const& ex)
{
// Details in ex.message() and ex.to_abi().
}
}
int main() {
init_apartment();
Uri uri{ L"http://www.google.com/"sv };
HttpClientExample(uri);
Sleep(10000);
}
How do i set a Custom Header using HttpFormUrlEncodedContent and how do i set custom content in the POST Body?
Thank you for your help!
application/x-www-form-urlencoded
<uri>?<key1>=<val1>&<key2>=<val2>&...
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
An
application/x-www-form-urlencoded
POST request sends its content as part of the URL (as opposed to the body), as a list of key-value pairs (<uri>?<key1>=<val1>&<key2>=<val2>&...
). You can set up a HttpFormUrlEncodedContent object by passing the key-value pairs into its constructor. You can assign headers by accessing its Headers property.– IInspectable
16 hours ago