Use sychronized or not below code generating same hashcode for all threads. How?
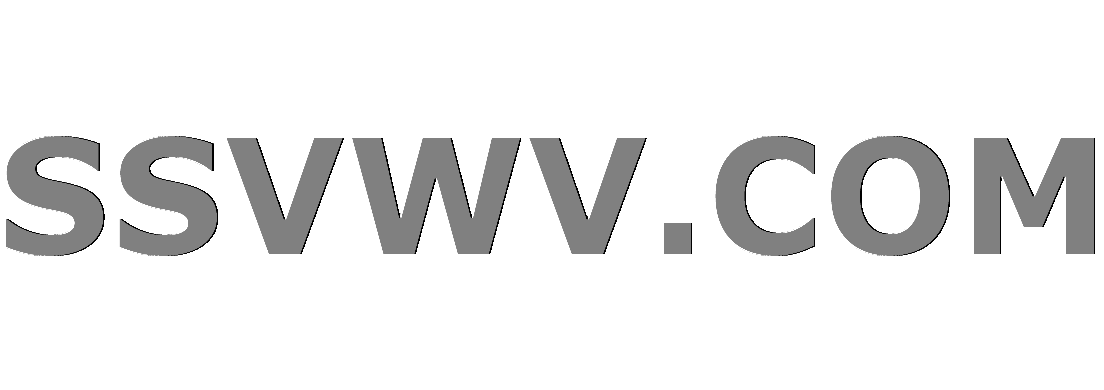
Multi tool use
Use sychronized or not below code generating same hashcode for all threads. How?
"we make the global access method synchronized so that only one thread can execute getInstance method at a time" without using synchronized keyword below code acting like threadsafe already.
Car.java
public class Car {
private static Car car;
private Car() {
// TODO Auto-generated constructor stub
}
public static Car getInstance(){
if(car==null){
car=new Car();
}
return car;
}
}
Test.java
public class Test {
public static void main(String args) {
Thread t1=new Thread(new Runnable() {
@Override
public void run() {
System.out.println(Thread.currentThread().getName()+" Running");
System.out.println(Car.getInstance().hashCode());
System.out.println(Thread.currentThread().getName()+" Finishing");
}
});
t1.start();
Thread t2=new Thread(new Runnable() {
@Override
public void run() {
System.out.println(Thread.currentThread().getName()+" Running");
System.out.println(Car.getInstance().hashCode());
System.out.println(Thread.currentThread().getName()+" Finishing");
}
});
t2.start();
Thread t3=new Thread(new Runnable() {
@Override
public void run() {
System.out.println(Thread.currentThread().getName()+" Running");
System.out.println(Car.getInstance().hashCode());
System.out.println(Thread.currentThread().getName()+" Finishing");
}
});
t3.start();
}
}
Output
Thread-0 Running
1828451009
Thread-0 Finishing
Thread-1 Running
1828451009
Thread-1 Finishing
Thread-2 Running
1828451009
Thread-2 Finishing
Without using synchronized method hashcode are same in all thread case, how?
t1.start(); t2.start(); t3.start();
tried this too but still unexpected output coming
– Rushikesh Maliye
Jul 1 at 11:25
2 Answers
2
If i start all threads at a time
t1.start();
t2.start();
t3.start();
output is varying sometime same hashcode sometimes its different
Thread-1 Running
Thread-2 Running
Thread-0 Running
183297081
Thread-2 Finishing
183297081
Thread-1 Finishing
183297081
Thread-0 Finishing
Thread-0 Running
Thread-2 Running
Thread-1 Running
678111939
1828451009
1828451009
Thread-2 Finishing
Thread-1 Finishing
Thread-0 Finishing
Thread-0 Running
Thread-2 Running
Thread-1 Running
678111939
183297081
558529301
Thread-1 Finishing
Thread-2 Finishing
Thread-0 Finishing
Unable to understand this behaviour
That is EXACTLY what is expected: non-reproducible, weird, "unexpected" output. What more reason do you need to see that your code is not thread-safe?
– luk2302
Jul 1 at 11:26
yes got it, to maintain consistency in output we need synchronized method in singleton case. thanks @luk2302
– Rushikesh Maliye
Jul 1 at 11:29
This is not a forum and answers are not used to elaborate on your question. Please edit your question instead.
– Nathan Hughes
Jul 1 at 11:56
Thread scheduling is part of OS, if you execute the code several times you find differences. If you want your code thread safe in all cases then you have to use synchroized block with double locking.
public class Car {
private static volatile Car car;
private Car() {
// TODO Auto-generated constructor stub
}
public static Car getInstance(){
if(car==null){
synchronized(Car.class) {
if(car==null)
car=new Car();
}
}
return car;
}
}
Note : Defining the whole method synchronized having performance
issue.
https://javarevisited.blogspot.com/2014/05/double-checked-locking-on-singleton-in-java.html
ok thanks for answering, got this point
– Rushikesh Maliye
Jul 1 at 11:35
Happy to help :) Mark it correct if it works with you
– Gaurav Srivastav
Jul 1 at 11:36
This code is not thread safe. This is a classic DCL bug.
– Boris the Spider
Jul 1 at 11:40
volatile keyword could you used to avoid thread caching and written to main memory.
– Gaurav Srivastav
Jul 1 at 11:52
If you were to read the article, written by none other than Goetz, you'll find that this doesn't help unless every field in
Car
is also volatile
. This answer is just wrong.– Boris the Spider
Jul 2 at 6:12
Car
volatile
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
That is the hard part of thread safety: even thread-unsafe code may seem thread-safe if you observe it the wrong way. In your case the threads are simply executed one after another, there is no real multi threading happening at all in your case. If you write
t1.start(); t2.start(); t3.start();
you may see a different result.– luk2302
Jul 1 at 11:11