Android Obtain all the children that a Layout has (Including Sub-childs)
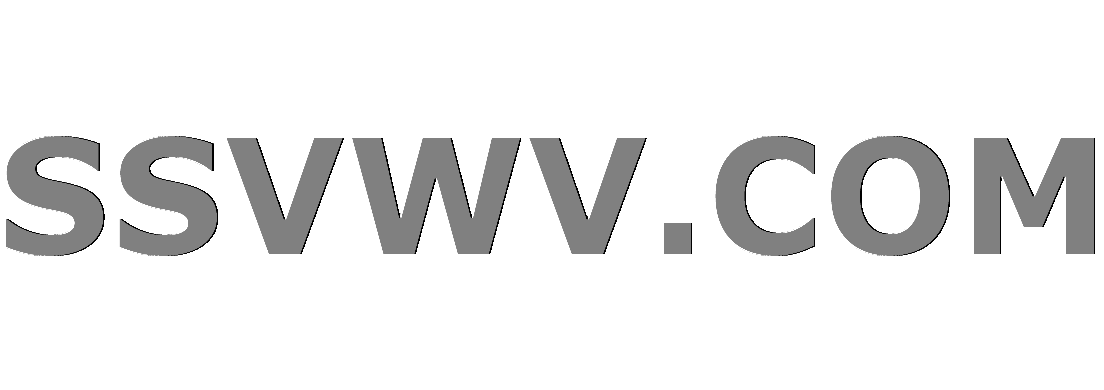
Multi tool use
Android Obtain all the children that a Layout has (Including Sub-childs)
Basically what im trying to do is create a function that has a recursive calling, so i can obtain all the RadioButtons, Buttons, FAB, TextView, and even others SubViews ( LinearLayouts, RelativeLayouts, FrameLayout, ViewGroup ). Right now i can obtain all the Layouts but when i wanna access the subcontent of those subViews those values never return...
var contentList = ArrayList<String>()
fun ManageView(viewGroup: ViewGroup, action: String, context: Activity){
try {
loop@ for (i in 0 until viewGroup.childCount) {
var child = viewGroup.getChildAt(i)
contentList.add(context.applicationContext.resources.getResourceEntryName(child.id))
when (child) {
is ViewGroup->{
ManageView(child,"vacant",context)
}
is RadioGroup -> {
contentList.add(context.applicationContext.resources.getResourceEntryName(child.id))
}
}
}
println("TOTAL OF ELEMENTS: " + contentList.size)
contentList.forEach { list ->
println("Data: " + list)
}
}catch (exception: Exception){
println("Houston we have a layout problem")
}
}
and the values that i got are:
07-01 07:35:40.543 30090-30090/com.epictech.epiccab_driver I/System.out: TOTAL OF ELEMENTS: 18
Data: framePrice
Data: lblRate
Data: viewInclude
Data: layoutPaidHired
Data: viewSeparator
Data: idPaidSign
Data: txtPaidResult
Data: viewSeparatorRight
Data: containerHiredDetails
Data: layoutHiredPays
Data: viewSeparatorPaidRight
Data: frameTripRateInfo
07-01 07:35:40.544 30090-30090/com.epictech.epiccab_driver I/System.out: Data: fragment_fragment_trip_info
Data: frameContainer
Data: layoutDriverData
Data: menu
Data: layoutFAB
Data: layoutSpeedometer
However in my xml i got a lot of Content that has a mix of FrameLayout, Relative and Linear. This is an example:
MainActivity
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/mainView"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@color/transparent"
tools:context="com.epictech.epiccab_driver.UI.MainActivity"
android:keepScreenOn="true">
<RelativeLayout
android:id="@+id/framePrice"
android:layout_width="510dp"
android:layout_height="wrap_content"
android:visibility="gone"
android:layout_toEndOf="@+id/layoutDriverData">
<TextView
android:id="@+id/lblRate"
android:layout_width="match_parent"
android:layout_height="27dp"
android:gravity="start|center_vertical"
android:paddingStart="10dp"
android:paddingEnd="10dp"
android:background="?attr/colorPrimary"
android:textColor="@color/md_black_1000"
android:textSize="12sp"
android:text="@string/activity_main_title_rate" />
<include
android:id="@+id/viewInclude"
android:layout_width="wrap_content"
android:layout_height="100dp"
android:layout_below="@+id/lblRate"
layout="@layout/fragment_paid_hired" />
</RelativeLayout>
<RelativeLayout
android:id="@+id/frameTripRateInfo"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:background="?attr/colorPrimaryDark"
android:visibility="gone"
android:layout_toEndOf="@+id/framePrice"
android:baselineAligned="false">
<include
android:layout_width="wrap_content"
android:layout_height="wrap_content"
layout="@layout/fragment_fragment_hired_trip_info" />
</RelativeLayout>
<FrameLayout
android:id="@+id/frameContainer"
android:layout_width="match_parent"
android:layout_above="@+id/menu"
android:layout_height="match_parent"
android:layout_alignParentEnd="true"
android:layout_below="@+id/frameTripRateInfo"
android:layout_toEndOf="@+id/layoutDriverData">
</FrameLayout>
<RelativeLayout
android:id="@+id/layoutDriverData"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:background="?attr/backgroundColor"
android:layout_above="@+id/menu">
<include
layout="@layout/card_driver_status"/>
</RelativeLayout>
<RelativeLayout
android:id="@+id/menu"
android:layout_width="match_parent"
android:layout_height="80dp"
android:layout_alignParentBottom="true">
<include
layout="@layout/menu_layout"/>
</RelativeLayout>
<RelativeLayout
android:id="@+id/layoutFAB"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_below="@+id/layoutSpeedometer"
android:layout_above="@+id/menu"
android:layout_alignParentEnd="true">
<include
layout="@layout/fab_map"/>
</RelativeLayout>
<RelativeLayout
android:id="@+id/layoutSpeedometer"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentEnd="true">
</RelativeLayout>
</RelativeLayout>
and this is the MenuLayout that MainActivity include
MenuLayout
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="80dp">
<FrameLayout
android:id="@+id/frameLayouTripInfo"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:visibility="gone">
<include layout="@layout/fragment_trip_info" />
</FrameLayout>
<View
android:id="@+id/viewSeparatorTop"
android:layout_width="match_parent"
android:layout_height="1dp"
android:layout_below="@+id/frameLayouTripInfo"
android:background="@color/md_white_1000" />
<RadioGroup
android:id="@+id/menuGroup"
android:layout_width="match_parent"
android:layout_height="80dp"
android:layout_below="@+id/viewSeparatorTop"
android:orientation="horizontal"
android:background="?attr/backgroundColor"
android:weightSum="1"
android:divider="@drawable/divider"
android:dividerPadding="7dip"
android:showDividers="middle">
<RadioButton
android:id="@+id/radioBtnStartTrip"
style="@style/RadioButtonState"
android:layout_width="wrap_content"
android:layout_height="80dp"
android:layout_weight="0.14285714"
android:text="@string/start_trip" />
<RadioButton
android:id="@+id/radioBtnAddToll"
style="@style/RadioButtonState"
android:layout_width="wrap_content"
android:layout_height="80dp"
android:layout_weight="0.14285714"
android:text="@string/add_toll" />
<RadioButton
android:id="@+id/radioBtnAddExtras"
style="@style/RadioButtonState"
android:layout_width="wrap_content"
android:layout_height="80dp"
android:layout_weight="0.14285714"
android:text="@string/add_extras" />
<RadioButton
android:id="@+id/radioBtnOffDuty"
style="@style/RadioButtonState"
android:layout_width="wrap_content"
android:layout_height="80dp"
android:layout_weight="0.14285714"
android:text="@string/off_duty" />
<LinearLayout
android:id="@+id/emailBtn"
android:layout_width="wrap_content"
android:layout_height="80dp"
android:layout_weight="0.14285714"
android:layout_gravity="center"
android:gravity="center"
android:padding="9dp"
android:background="?attr/backgroundColor">
<RelativeLayout
android:id="@+id/layoutEmail"
android:layout_width="100dp"
android:layout_height="80dp"
android:background="@drawable/email"
android:backgroundTint="@color/md_black_1000"
android:layout_gravity="center">
<com.epictech.epiccab_driver.Helper.Notification.CounterTextView
android:id="@+id/notification_count"
android:layout_width="wrap_content"
android:layout_height="match_parent"
android:layout_alignParentEnd="true"
android:layout_alignParentTop="true"
android:layout_marginTop="19dp"
android:gravity="center"
android:paddingBottom="2dp"
android:paddingLeft="4dp"
android:paddingRight="4dp"
android:paddingTop="22dp"
android:textColor="@android:color/white"
android:textSize="20sp"
app:ctv_rounded_background_color="@color/md_red_600"
tools:text="99+" />
</RelativeLayout>
</LinearLayout>
<ImageView
android:id="@+id/settings"
android:layout_width="wrap_content"
android:layout_height="80dp"
app:srcCompat="@drawable/settings"
android:padding="7dp"
android:backgroundTint="?attr/primaryTextColor"
android:layout_gravity="center"
android:scaleType="fitCenter"
android:layout_weight="0.14285714"
android:contentDescription="@string/configurations" />
<RadioButton
android:id="@+id/radioBtnEndShift"
style="@style/RadioButtonState"
android:layout_width="wrap_content"
android:layout_height="80dp"
android:layout_weight="0.14285714"
android:text="@string/end_shift" />
</RadioGroup>
</RelativeLayout>
Please tell me what im doing wrong...
Thanks for all the support!!
Yeah, all the views and the content that those views has ( EditText, InputText, RadioButton, etc )
– Luis Cardoza Bird
Jul 1 at 14:08
From your example you're collecting only RadioGroup so I added an answer for that case.
– user8035311
Jul 1 at 14:11
1 Answer
1
To collect all the RadioButton
views(as specified in your example) just change your code as follows. To collect more types put them in the same when
block:
RadioButton
when
var contentList = ArrayList<String>()
fun ManageView(view: View, action: String, context: Activity) {
try {
// if you want all the views then add elements to the list without any when
when (view) {
is RadioGroup -> {
loop@ for (j in 0 until view.childCount){
val child = view.getChildAt(j)
contentList.add(context.applicationContext.resources.getResourceEntryName(child.id))
}
}
//add more cases here to collect more types
// all the view types that you'd like to collect must be put here
}
println("TOTAL OF ELEMENTS: " + contentList.size)
contentList.forEach { list ->
println("Data: " + list)
}
if (view is ViewGroup) {
loop@ for (i in 0 until view.childCount) {
val child = view.getChildAt(i)
when (child) {
is ViewGroup -> {
ManageView(child, "vacant", context)
}
}
}
}
}catch (exception: Exception){
println("Houston we have a layout problem")
}
}
The result is the same, but now i cannot obtain the other views. TOTAL OF ELEMENTS: 1 Data: menuGroup
– Luis Cardoza Bird
Jul 1 at 14:43
@LuisCardozaBird I forgot to move println out of the if. I edited the answer.
– user8035311
Jul 1 at 14:45
Still the value is the same, the problem is that the function isn't getting the value assigned, the missing thing is the loop in the first when case
– Luis Cardoza Bird
Jul 1 at 14:50
I edited the answer, now i can obtain the RadioButtons :) thanks
– Luis Cardoza Bird
Jul 1 at 14:52
You;'re welcome :)
– user8035311
Jul 1 at 15:09
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Are you trying to get all the views?
– user8035311
Jul 1 at 14:07