Get partial class name and text inside div using regex
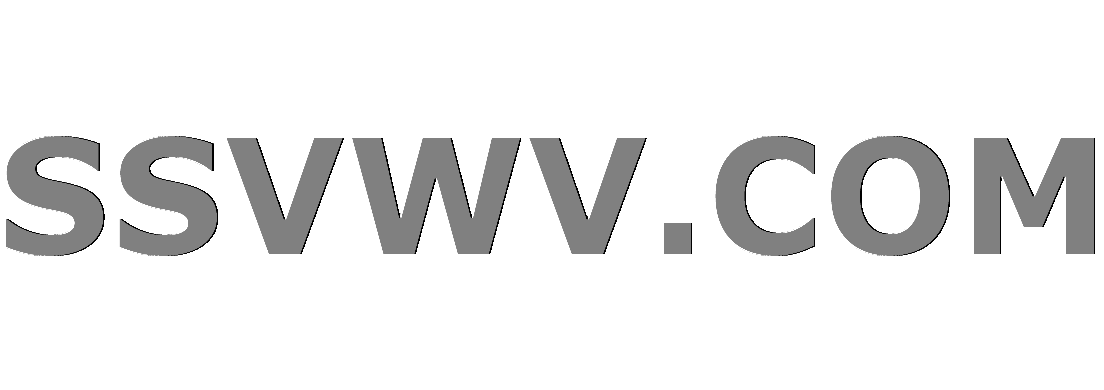
Multi tool use
Get partial class name and text inside div using regex
I need to get two values from this HTML, which is either an error or a success from toast-*
, and also get the value inside the toast-message
:
toast-*
toast-message
<div class="toast toast-error" style="">
<div class="toast-message">You have failed.</div>
</div>
<div class="toast toast-success" style="">
<div class="toast-message">You have succeed.
</div>
The div
elements only show once at a single time, which can be either error or success.
div
Is there any way I can use regex, so I can extract the value within array so it either:
['success', 'You have succeed.']
or
['error', 'You have failed.']
Any help would be greatly appreciated. Thanks!
@CertainPerformance because that div in string format and not original html file
– bakaio
Jul 1 at 10:57
@bakaio And are you unable to parse the string as HTML? At with the other 1000 times per day that a "how do I parse HTML with regex" question appears on StackOverflow, I'll post the obligatory: TH̘Ë͖́̉ ͠P̯͍̭O̚N̐Y̡ H̸̡̪̯ͨ͊̽̅̾̎Ȩ̬̩̾͛ͪ̈́̀́͘ ̶̧̨̱̹̭̯ͧ̾ͬC̷̙̲̝͖ͭ̏ͥͮ͟Oͮ͏̮̪̝͍M̲̖͊̒ͪͩͬ̚̚͜Ȇ̴̟̟͙̞ͩ͌͝S̨̥̫͎̭ͯ̿̔̀ͅ
– Tom Lord
Jul 1 at 11:11
The "right" way to parse HTML is by using an HTML parser. Not a regex. For example, with Nokogiri. Is there definitely a good reason for you to use regex?
– Tom Lord
Jul 1 at 11:13
If you control the HTML generation and can guarantee that it will be of the exact same format every time -- same attribute order, same whitespace, no variation in the node structure, etc -- then you might be able to get away with doing this in regex. Of course, if you can guarantee that, then you probably don't need regex, because you could extract the data from whatever upstream process is generating the HTML in the first place.
– Daniel Beck
Jul 1 at 11:24
3 Answers
3
You might use a DOMParser and querySelector to select the elements.
let parser = new DOMParser();
let html = `<div class="toast toast-error" style=""><div class="toast-message">You have failed.</div></div>`;
let doc = parser.parseFromString(html, "text/html");
let div = doc.querySelector("div.toast");
let successOrFailure = div.getAttribute('class')
.split(" ")
.filter(word => word.substring(0, 6) === "toast-")[0]
.substring(6);
let message = div.firstChild.innerHTML;
let result = [successOrFailure, message];
console.log(result);
Thank you, I replace domparser with jsdom since its running on node.
– bakaio
Jul 1 at 12:27
A preferred way would be to use the source strings and, with the help of the DOM, test, whether the element is present:
const html1 = `
<div class="toast toast-error" style="">
<div class="toast-message">You have failed.</div>
</div>
`;
const html2 = `
<div class="toast toast-success" style="">
<div class="toast-message">You have succeed.</div>
</div>
`;
/**
* Returns the text of the error toast message.
*
* @returns {string|null} The toast message, or null if the elemenet is not present.
*/
function getErrorToastText(string) {
const container = document.createElement('div');
container.innerHTML = string;
const toast = container.querySelector('.toast-error .toast-message');
return toast ? toast.innerHTML : null;
}
/**
* Returns the text of the success toast message.
*
* @returns {string|null} The toast message, or null if the elemenet is not present.
*/
function getSuccessToastText(string) {
const container = document.createElement('div');
container.innerHTML = string;
const toast = container.querySelector('.toast-success .toast-message');
return toast ? toast.innerHTML : null;
}
console.log('when .error-toast element present:', getErrorToastText(html1));
console.log('when .error-toast element not present:', getErrorToastText(html2));
console.log('when .success-toast element present:', getSuccessToastText(html2));
console.log('when .success-toast element not present:', getSuccessToastText(html1));
There are many ways to do it. I only listed three of them, to see all of them check this answer
1. Using (ES6) JS function includes
includes
var str = "toast toast-success",
substr = "success";
str.includes(substr);
2. Using indexOf
indexOf
var str = "toast toast-success",
substr = "success";
if(str.indexOf(substr) == 1){
// the current div is a success
// do your logic
}
3. Using Regex
// you get this string by getting class-list of the div
var str= "toast toast-success",
substr= /-success$/; // or /-error$/
string.match(expr);
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Why do you want to use a regular expression here? Do you have a reference to the elements?
– CertainPerformance
Jul 1 at 10:53