Raspberry pi and arduino serial connection over usb displays USB information
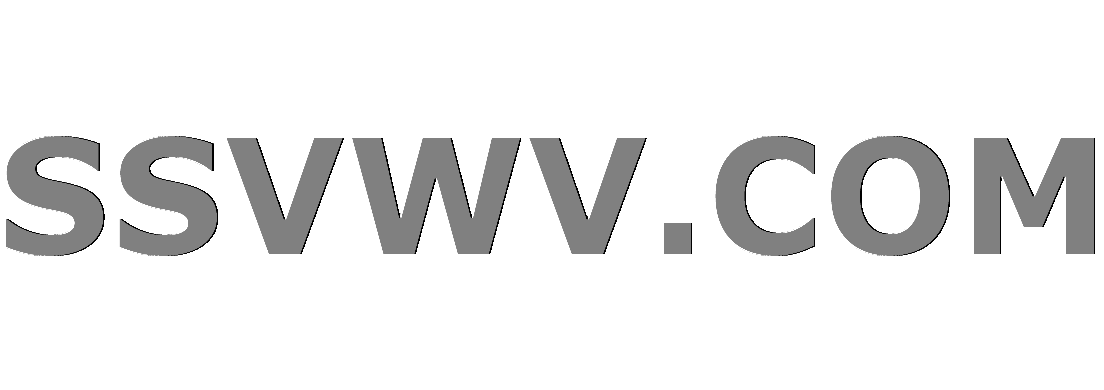
Multi tool use
Raspberry pi and arduino serial connection over usb displays USB information
I was trying to talk between the Arduino and raspberry pi using USB, with the pySerial library. I wanted to have multiple sensors sending in data and a couple of command sent from the pi's side. I've tried arrays to send data and use indexing on the python (receiving) side to access individual data channels, is there another way to do this task, I've tried learning about "data.split(':')", I don't seem to understand how it works. Could someone please show me a legal method to transfer data to-fro?
Arduino Code:
int data[100];
int val2 = A0;
int val1 = A1;
void setup(){
pinMode(val1, INPUT);
pinMode(val2, INPUT);
Serial.begin(9600);
}
void loop(){
// individual channels of data transmitted, delya = 60 for 6 channels, f = 1/50
while(1){
data[0] = analogRead(val2);
data[1] = analogRead(val1);
data[2] = analogRead(A2);
data[4] = analogRead(A3);
data[5] = analogRead(A4);
Serial.print(data[0]);
Serial.print(":");
Serial.print(data[1]);
Serial.print(":");
Serial.print(data[2]);
Serial.print(":");
Serial.print(data[3]);
Serial.print(":");
Serial.print(data[4]);
Serial.print(":");
Serial.print(data[5]);
Serial.println();
delay(60);
}
}
Python side:
import serial
from Tkinter import *
import time
data =
newData =
next =
root = Tk()
while True:
ardu = serial.Serial('/dev/ttyACM0', 9600, timeout = 0.1)
data =ardu.readline()
print data[0:25]
1 Answer
1
You are correct, you need Python's split()
method. You can use it as follows:
split()
int data[6];
int val2 = A0;
int val1 = A1;
void setup(){
pinMode(val1, INPUT);
pinMode(val2, INPUT);
Serial.begin(9600);
}
void loop(){
// individual channels of data transmitted, delay = 60 for 6 channels, f = 1/50
while(1){
data[0] = analogRead(val2);
data[1] = analogRead(val1);
data[2] = analogRead(A2);
data[3] = analogRead(A3);
data[4] = analogRead(A4);
data[5] = analogRead(A5);
Serial.print(data[0]);
Serial.print(":");
Serial.print(data[1]);
Serial.print(":");
Serial.print(data[2]);
Serial.print(":");
Serial.print(data[3]);
Serial.print(":");
Serial.print(data[4]);
Serial.print(":");
Serial.print(data[5]);
Serial.println();
delay(60);
}
}
import serial
ser = serial.Serial('/dev/ttyACM0', 9600)
while True:
line = ser.readline().decode("utf-8").strip('n').strip('r') # remove newline and carriage return characters
print "Received: '{}'".format(line)
data = line.split(':')
for i in range(len(data)):
print "data[{}]".format(i), data[i]
Received: '495:916:837:37:120'
data[0] 495
data[1] 916
data[2] 837
data[3] 37
data[4] 120
split()
creates a list from a string, the elements of the list are the parts of the string between two pairs of :
, or between the start/end and another :
.
split()
:
:
@PrathikGurudatt, you can only index it once you've used
split()
. Mark as accepted if it has helped you.– Morgoth
Mar 20 '17 at 0:20
split()
Thank-you, Morgoth - I was looking for the code to cleanup the Arduino print output received on the Pi side Your decode("utf-8").strip('n').strip('r') does a nice job succinctly :-) The split implementation is a bonus for me !
– user3902302
Jul 1 at 3:19
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
ahh i see, quite precise though :) But is it also possible to use the usual indexing method?
– Prathik Gurudatt
Mar 19 '17 at 15:32